arcgis engine 将读取属性表过程封装为一个有参数的方法体,传入参数类型为IFeatureLayer类型,方法名称自定义 代码
时间: 2024-10-18 15:18:35 浏览: 29
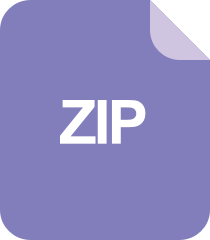
ArcGIS-Engine+C#-初学者实例代码.zip_ArcGISEngine_HWE7_arcgis engine_eng

ArcGIS Engine 提供了一种方便的方式来封装常见的操作,如读取属性表。你可以创建一个静态方法或者实例方法,接收 `IFeatureLayer` 类型的参数,这个类代表的是 ArcGIS 中的数据层对象。下面是一个简单的示例代码:
```csharp
using ESRI.ArcGIS.Geometry;
using ESRI.ArcGIS.Carto;
using ESRI.ArcGIS.Geodatabase;
public static class AttributeTableReader
{
// 自定义方法名,这里假设为 "ReadAttributes"
public static void ReadAttributes(IFeatureLayer featureLayer, string outputTableName)
{
// 检查输入是否有效
if (featureLayer == null)
throw new ArgumentNullException("featureLayer");
// 获取数据源
IFeatureClass featureClass = featureLayer.FeatureClass;
// 创建一个事务上下文
ITransaction transaction = new Transaction();
transaction.Start();
try
{
// 使用地理数据库连接打开表
IGeodatabase geodatabase = featureClass.Workspace as IGeodatabase;
ITable outputTable = geodatabase.CreateTable(outputTableName, featureClass.Name);
// 读取属性表
ICursor cursor = featureClass.Search(null, false);
while ((IFeature)cursor.Next() != null)
{
IRow row = outputTable.InsertRow();
// 遍历字段并设置值
foreach (IVariant field in cursor.Current.Fields)
{
row.SetField(field.Name, field.Value);
}
row.Store();
}
transaction.Commit();
}
catch (Exception ex)
{
transaction.Abort();
Console.WriteLine($"Error reading attributes: {ex.Message}");
throw;
}
finally
{
// 关闭游标和事务
if (cursor != null)
cursor.Close();
transaction.AbortOrCommit(); // 如果交易没提交,在finally里关闭它
}
}
}
```
使用该方法时,可以这样做:
```csharp
// 假设你已经有了一个 FeatureLayer 对象
IFeatureLayer myFeatureLayer = ...;
// 调用方法,传递需要的表名
AttributeTableReader.ReadAttributes(myFeatureLayer, "OutputTable");
```
阅读全文
相关推荐
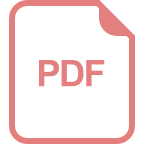
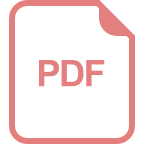
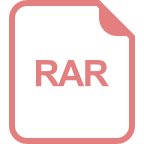
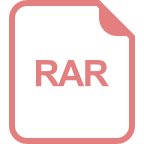
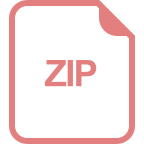
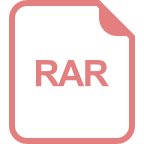
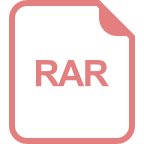
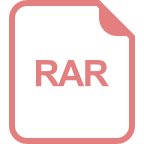
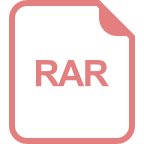
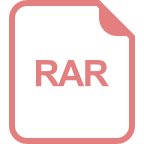
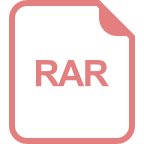
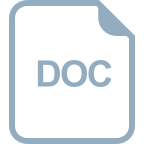
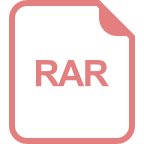
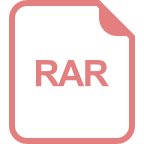
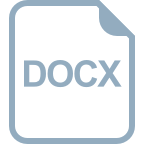
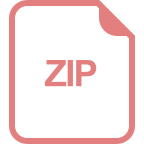
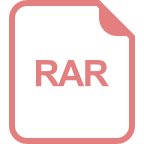