java数组实现队列代码
时间: 2024-10-01 14:03:08 浏览: 34
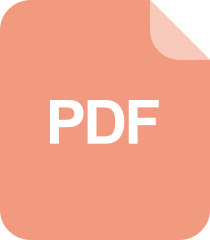
基于Java数组实现循环队列的两种方法小结
在Java中,可以使用数组实现一个简单的队列,基本思路是创建一个固定大小的数组,然后利用两个指针(front和rear)分别表示队头和队尾。以下是使用数组实现单端队列(先进先出,FIFO)的一个简单示例:
```java
public class ArrayQueue {
private int capacity;
private int[] queue;
private int front, rear;
public ArrayQueue(int size) {
capacity = size + 1; // 队列容量加1是因为需要一个额外的空间存储队尾元素
queue = new int[capacity];
front = -1;
rear = -1;
}
// 插入元素到队尾
public void enqueue(int value) {
if (isFull()) {
throw new RuntimeException("Queue is full");
}
if (front == -1) {
front = 0;
}
rear = (rear + 1) % capacity;
queue[rear] = value;
}
// 从队头移除并返回元素
public int dequeue() {
if (isEmpty()) {
throw new RuntimeException("Queue is empty");
}
int removedValue = queue[front];
if (front == rear) {
front = rear = -1;
} else {
front = (front + 1) % capacity;
}
return removedValue;
}
// 判断队列是否为空
public boolean isEmpty() {
return front == -1;
}
// 判断队列是否满
public boolean isFull() {
return (rear + 1) % capacity == front;
}
}
```
在这个例子中,`enqueue()`方法将元素添加到队尾,`dequeue()`方法从队头移除元素,并有适当的异常处理防止错误的操作(如尝试从空队列中删除元素或往已满的队列中插入)。注意队列的索引是从0开始计数的,所以当后端指针到达最大值时,它会从0开始循环。
阅读全文
相关推荐
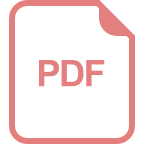
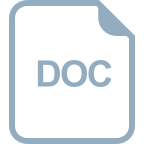
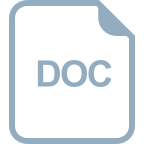
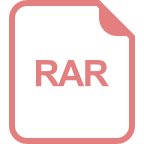
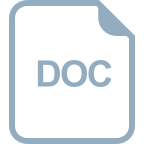
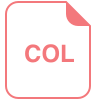

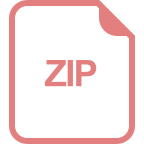
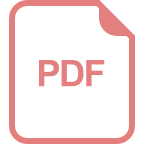
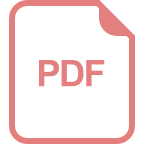
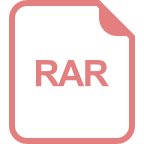
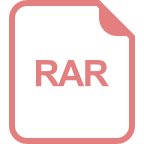
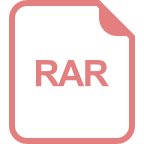
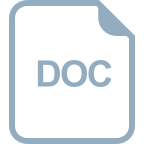
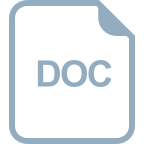
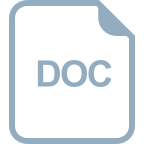
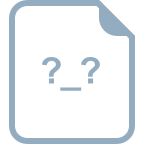