Linux系统,用c语言写一段代码,实现对文件加解密
时间: 2024-12-12 09:31:47 浏览: 4
Linux系统中使用C语言实现简单的文件加密和解密通常会涉及到基本的文件操作、字符串处理以及常见的加密算法,如Caesar Cipher(凯撒密码)或更复杂的像AES(高级加密标准)。这里我将提供一个简单的凯撒密码示例,用于对文本文件进行加密和解密。请注意,这是一种非常基础的加密方法,不适合存储敏感信息。
首先,我们需要创建两个函数,一个用于加密,一个用于解密:
```c
#include <stdio.h>
#include <string.h>
// 加密函数,字符向右移动指定位数
char caesar_encrypt(char ch, int shift) {
if (ch >= 'a' && ch <= 'z') {
return ((ch - 'a' + shift) % 26) + 'a';
} else if (ch >= 'A' && ch <= 'Z') {
return ((ch - 'A' + shift) % 26) + 'A';
}
return ch; // 非字母字符不变
}
// 解密函数,恢复到原始位置
char caesar_decrypt(char ch, int shift) {
return caesar_encrypt(ch, -shift);
}
void encrypt_file(const char* input_path, const char* output_path, int shift) {
FILE* fin = fopen(input_path, "r");
FILE* fout = fopen(output_path, "w");
if (!fin || !fout) {
perror("Error opening file");
return;
}
char buffer[1024];
while (fgets(buffer, sizeof(buffer), fin)) {
for (int i = 0; buffer[i] != '\n'; i++) {
buffer[i] = caesar_encrypt(buffer[i], shift);
}
fputs(buffer, fout);
}
fclose(fin);
fclose(fout);
printf("File encrypted to: %s\n", output_path);
}
void decrypt_file(const char* input_path, const char* output_path, int shift) {
FILE* fin = fopen(input_path, "r");
FILE* fout = fopen(output_path, "w");
if (!fin || !fout) {
perror("Error opening file");
return;
}
char buffer[1024];
while (fgets(buffer, sizeof(buffer), fin)) {
for (int i = 0; buffer[i] != '\n'; i++) {
buffer[i] = caesar_decrypt(buffer[i], shift);
}
fputs(buffer, fout);
}
fclose(fin);
fclose(fout);
printf("File decrypted to: %s\n", output_path);
}
// 使用示例
int main() {
int shift = 3; // 加密偏移量
encrypt_file("input.txt", "encrypted.txt", shift);
decrypt_file("encrypted.txt", "decrypted.txt", shift);
return 0;
}
```
在这个例子中,`encrypt_file()` 和 `decrypt_file()` 函数分别用于加密和解密文件。你可以替换 `input.txt` 和 `output.txt` 为实际的文件路径,以及 `shift` 变量来改变加密强度。
阅读全文
相关推荐




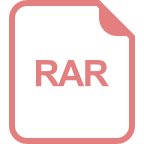




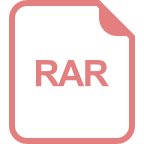








