c++输入一个日期,输出明天的日期,考虑所有的可能
时间: 2024-10-17 11:04:35 浏览: 69
在C++中,处理日期通常需要使用一些库,如C++11引入的`<chrono>`头文件,或者第三方库如`date.h`。这里我会给出一个基本的实现,使用`<chrono>`和`<ctime>`来获取和操作当前日期,并添加一天。请注意,这个例子假设用户输入的是一个合法的日期格式:
```cpp
#include <iostream>
#include <ctime>
#include <chrono>
void getTomorrowDate(const std::string& input_date) {
// 解析日期,这里简单地假定格式为 "YYYY-MM-DD"
std::tm parsed_date = {};
std::istringstream iss(input_date);
iss >> std::get_time(&parsed_date, "%Y-%m-%d");
// 创建时间点对象,基于解析后的日期和本地时间
auto local_time = std::chrono::time_point<std::chrono::system_clock, std::chrono::seconds>(
std::chrono::hours(parsed_date.tm_hour),
std::chrono::minutes(parsed_date.tm_min),
std::chrono::seconds(parsed_date.tm_sec));
// 添加一天
auto tomorrow = local_time + std::chrono::days(1);
// 获取明天的日期,格式化输出
auto tomorrow_day = std::chrono::system_clock::to_time_t(tomorrow);
std::cout << std::ctime(&tomorrow_day) << "\n";
}
int main() {
std::string input_date;
std::cout << "请输入日期(格式:YYYY-MM-DD): ";
std::cin >> input_date;
getTomorrowDate(input_date);
return 0;
}
```
此代码会提示用户输入日期,然后输出明天的日期。由于`std::get_time`函数对日期格式比较敏感,如果输入的日期格式错误,程序可能会崩溃。在实际应用中,你可能需要更复杂的日期解析和验证机制。
阅读全文
相关推荐









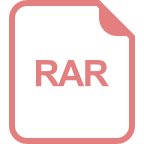







