有没有将字符串转为double类型的方法
时间: 2024-09-14 21:11:59 浏览: 42
在许多编程语言中,都有将字符串转换为double类型的方法。以下是一些常见编程语言的示例:
在Python中,可以使用`float()`函数将字符串转换为浮点数(即double类型)。例如:
```python
string_value = "3.14"
double_value = float(string_value)
```
在Java中,可以使用`Double.parseDouble()`方法将字符串转换为double类型。例如:
```java
String stringValue = "3.14";
double doubleValue = Double.parseDouble(stringValue);
```
在C++中,可以使用`atof()`函数将字符串转换为double类型。例如:
```c++
#include <cstdlib> // 需要包含此头文件才能使用atof函数
std::string stringValue = "3.14";
double doubleValue = atof(stringValue.c_str());
```
请注意,这些方法会将字符串转换为浮点数(double类型),如果字符串包含无法解析的数字或非数字字符,则转换可能会失败或产生错误。因此,在进行转换之前,最好确保字符串只包含有效的数字字符。
相关问题
C+=将字符串转换为double类型
C++ 中可以使用 `std::stod` 或 `std::to_string` 和 `std::atof` 函数来实现字符串到 double 类型的转换。具体操作步骤如下:
使用 `std::stod` 或 `std::to_string` 将字符串转换为 double 类型字符串,然后再使用 `std::atof` 函数将字符串转换为 double 类型。
示例代码:
```cpp
#include <iostream>
#include <string>
#include <cmath>
int main() {
std::string str = "3.14159";
double d = std::stod(str); // 使用 std::stod 将字符串转换为 double 类型
std::cout << d << std::endl; // 输出结果:3.14159
// 或者使用 std::to_string 和 std::atof 转换
str = "3.14159";
std::string str_double = std::to_string(std::atof(str)); // 使用 std::to_string 和 std::atof 将字符串转换为 double 类型字符串
d = std::stod(str_double); // 使用 std::stod 将字符串转换为 double 类型
std::cout << d << std::endl; // 输出结果:3.14159
return 0;
}
```
注意:在使用 `std::stod` 或 `std::to_string` 进行转换时,如果输入的字符串不能完全表示为一个有效的 double 类型数值,程序会抛出异常或产生不可预测的结果。因此,建议在使用这些函数进行转换时进行错误处理,确保输入的字符串符合要求。
将字符串转换为double类型呢
要将字符串转换为double类型,可以使用C++标准库中的stod函数。stod函数接受一个表示浮点数的字符串作为参数,并返回对应的double值。
下面是一个示例:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "3.14";
double num = std::stod(str);
std::cout << num << std::endl; // 输出:3.14
return 0;
}
```
与stoi函数类似,如果字符串无法转换为double类型,stod函数也会抛出`std::invalid_argument`或`std::out_of_range`异常。
需要注意的是,stod函数只能将字符串转换为double类型,如果需要将字符串转换为其他浮点类型(如float),则可以使用相应的函数(如stof)。
如果你有任何关于字符串转换为double类型的更具体问题,请随时提问!
阅读全文
相关推荐
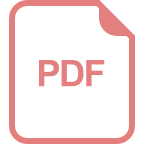
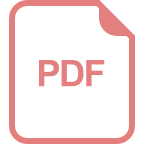
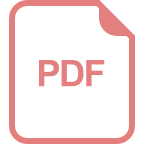













