给出公元元年到公元 3000年之间的一个年份,判断是否闰年,要求对输入的年份进行合法 性判断。
时间: 2024-10-14 10:15:57 浏览: 40
判断一个年份是否为闰年的标准通常基于格里高利历的规定,即闰年满足以下条件之一:
1. 能被4整除的年份是闰年,例如2004年、2020年;
2. 但是能被100整除的年份不是闰年,比如1900年就不是闰年;
3. 然而,如果一个年份同时能被400整除,它仍然是闰年,例如2000年就是闰年。
对于给定的公元元年到公元3000年之间的任何一个年份,我们可以编写一个简单的程序来进行检查。首先需要确保年份在范围内(从1到3000),然后应用上述规则。下面是一个基本的算法:
```python
def is_leap_year(year):
if year < 1 or year > 3000:
return False # 年份不在指定范围内
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0): # 格式化判断闰年条件
return True
else:
return False
# 测试示例
print(is_leap_year(2000)) # 输出:True
print(is_leap_year(1900)) # 输出:False
```
相关问题
给出公元元年到公元3000年之间的一个年份,判断是否闻年,要求对输入的年份进行合法性判断。用c语言表达
在 C 语言中,你可以通过创建一个函数来实现这个功能。首先,你需要检查输入的年份是否满足闰年的规则,然后判断它是否在公元元年至公元3000年之间。以下是简单的 C 代码示例:
```c
#include <stdbool.h>
// 判断是否为闰年
bool isLeapYear(int year) {
if (year % 4 != 0)
return false;
else if (year % 100 != 0)
return true;
else if (year % 400 != 0)
return false;
else
return true;
}
// 检查年份的有效性
bool isValidYear(int year, int startYear, int endYear) {
if (year >= startYear && year <= endYear) {
return isLeapYear(year);
} else {
return false; // 年份不在指定范围内
}
}
int main() {
int inputYear = 2000; // 用户输入的年份
int startDate = 1; // 公元元年
int endDate = 3000; // 公元3000年
if (isValidYear(inputYear, startDate, endDate)) {
printf("%d年是合法的,并且是闰年。\n", inputYear);
} else {
printf("%d年是非法的,或者不是闰年。\n", inputYear);
}
return 0;
}
```
在这个程序中,`isLeapYear` 函数用于判断给定的年份是否为闰年,`isValidYear` 函数则会检查年份是否在指定范围并同时是闰年。在 `main` 函数中,你可以将 `inputYear` 替换为你想测试的具体年份。
用户输入一个公元纪年的年月日形式,计算从公元元年开始,到用户输入的年份为止的天数、小时数、分钟数和秒数。(请注意闰年)
计算从公元元年到指定日期的总天数、小时数、分钟数和秒数需要考虑闰年规则。首先,我们将按照每年365天(平年)和366天(闰年)来进行计算。步骤如下:
1. **计算总天数**:
- 对于每个非闰年,加1天。
- 对于闰年,判断是否能被4整除且不能被100整除,或者可以被400整除。如果是,则加1天。
2. **计算总小时数**:
将总天数乘以每天24小时。
3. **计算总分钟数**:
再将总小时数乘以每小时60分钟。
4. **计算总秒数**:
最后,将总分钟数乘以每分钟60秒。
为了精确计算,你可以创建一个函数,接受年、月、日作为输入,并遍历每个年份,检查闰年。以下是伪代码示例:
```python
def count_seconds(year, month, day):
days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
# 如果是闰年,二月增加一天
if is_leap_year(year):
days_in_month[1] += 1
total_days = sum(days_in_month[:month]) + day
total_hours = total_days * 24
total_minutes = total_hours * 60
total_seconds = total_minutes * 60
return total_seconds, total_minutes, total_hours, total_days
# 判断是否为闰年
def is_leap_year(year):
if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0):
return True
else:
return False
# 用户输入年月日
input_year = int(input("请输入年份:"))
input_month = int(input("请输入月份:"))
input_day = int(input("请输入日期:"))
seconds, minutes, hours, days = count_seconds(input_year, input_month, input_day)
```
在这个例子中,你需要编写`is_leap_year()`函数来准确处理闰年条件。
阅读全文
相关推荐

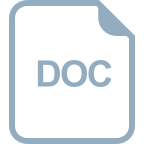




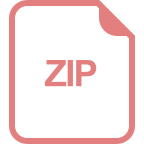








