帮我编写yoloe的predict.py推理程序,要求输出格式为detect_result={"category":"6010001","num":15}的格式,并绘制标好框的图片
时间: 2024-10-23 07:09:47 浏览: 26
在YOLOv5(You Only Look Once v5)的预测脚本`predict.py`中,你需要先加载模型、设置输出格式,并读取输入图像。下面是一个基本的示例,展示了如何实现你的需求:
```python
import torch
from PIL import Image
from models.experimental import attempt_load
from utils.datasets import LoadImages
from utils.general import non_max_suppression, apply_classifier, scale_coords
def yolov5_predict(image_path, model_path):
# 加载模型
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model = attempt_load(model_path, map_location=device)
# 设置输出格式
def predict_image(img):
img = Image.open(img)
img = preprocess_image(img) # 自定义预处理函数,通常包含resize和to_tensor
img = img.unsqueeze(0).to(device) # 添加通道维度并转到设备上
pred = model(img) # 运行预测
pred = non_max_suppression(pred, conf_thres=0.5, iou_thres=0.4) # NMS
boxes, labels, scores = pred[:, :4], pred[:, 5:].argmax(-1), pred[:, 4] # 分别提取坐标、类别标签和置信度
return [{"category": str(labels[i]), "num": int(scores[i].item()), "bbox": boxes[i]} for i in range(len(labels))]
detect_result = predict_image(image_path)
return detect_result
def draw_boxes(image_path, detect_result):
# 绘制边界框的图片,这里仅作演示,需要安装如matplotlib库并根据实际需求定制
import matplotlib.pyplot as plt
img = Image.open(image_path)
for result in detect_result:
bbox = result["bbox"]
category = result["category"]
num = result["num"]
# 使用bbox coordinates and the image to draw bounding box with label
# ... (实现具体的绘图逻辑)
plt.imshow(img)
plt.show()
# 使用示例
image_path = "path/to/image.jpg"
model_path = "path/to/yolov5s.pt" # 根据你的YOLOv5模型路径替换
detect_result = yolov5_predict(image_path, model_path)
draw_boxes(image_path, detect_result)
```
这个示例假设你已经对YOLOv5进行了预训练,并有一个预处理图像的函数`preprocess_image()`。对于绘制边框的部分,由于涉及到绘图库的选择和布局细节,这里提供了一个简单的框架,你需要根据实际使用的绘图库添加相应的代码。
阅读全文
相关推荐
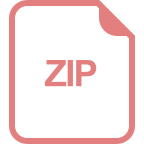
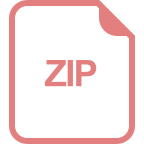

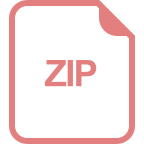
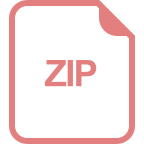
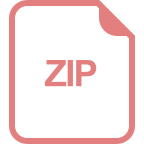
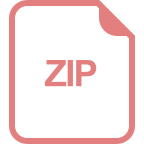
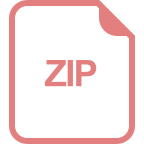
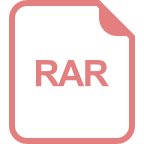
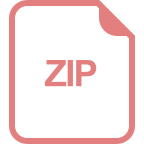
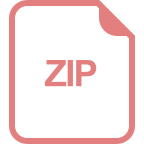
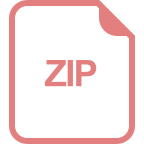
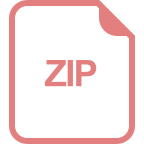
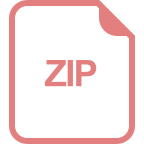