在platformio中写一个ESP32发送消息到企业微信应用的函数
时间: 2024-09-26 12:06:03 浏览: 35
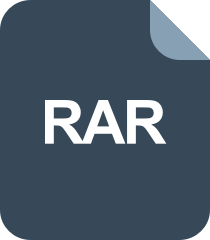
ESP32基于VScode_PlatformIO_发送邮件源码

在PlatformIO中编写一个ESP32设备通过微信企业号API发送消息的函数,首先需要安装相关的库和设置环境。以下是一个基本的示例,我们将使用`esphome`平台以及一个第三方库`esp32mqtt`(用于处理MQTT通信),因为企业微信的消息推送通常通过MQTT协议。
```cpp
#include <WiFi.h>
#include <WiFiClientSecure.h>
#include "mqttsn/mqttsn.h"
#include <json/json.hpp>
// 企业微信配置信息
const char* corpId = "your_corp_id";
const char* appSecret = "your_app_secret";
const char* agentId = "your_agent_id";
const char* mqttServer = "your_mqtt_server";
const int mqttPort = 443; // 使用HTTPS默认端口
WiFiClientSecure client;
MQTTSN_Client espMqtt(client);
void sendMessage(const String& toUser, const String& message) {
// 验证并连接到MQTT服务器
if (!espMqtt.connectToHost(mqttServer, mqttPort)) {
Serial.println("Failed to connect to MQTT server");
return;
}
// 创建一个JSON消息结构体
json_obj root;
json_obj msg;
msg.set("touser", toUser.c_str());
msg.set("msgtype", "text");
json_obj text;
text.set("content", message.c_str());
msg.add(json_obj::value_type("text", text));
// 将JSON编码为字符串
String encodedMsg = "";
try {
encodedMsg = json_obj_to_json_string(root);
} catch (std::exception &e) {
Serial.println(e.what());
return;
}
// 发送消息到企业微信指定主题
String topic = "wxapp/" + corpId + "/" + agentId + "/message/send";
// 编码为二进制发送
uint8_t payload[encodedMsg.length() + 1];
strncpy(payload, encodedMsg.c_str(), encodedMsg.length());
payload[encodedMsg.length()] = '\0';
size_t result = espMqtt.publish(topic, (uint8_t*)payload, encodedMsg.length());
// 如果发布成功
if (result == ESP_OK) {
Serial.println("Message sent successfully");
} else {
Serial.println("Failed to send message");
}
}
// ...其他主程序代码...
void setup() {
// WiFi和MQTT连接初始化...
}
void loop() {
// 检查网络连接和MQTT状态...
if (espMqtt.connected()) {
// 定义要发送消息的对象和内容...
sendMessage("toUser@example.com", "Hello from ESP32");
}
}
```
阅读全文
相关推荐
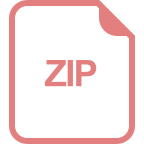
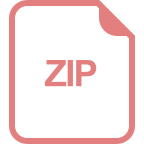
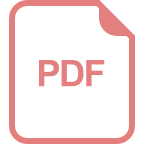
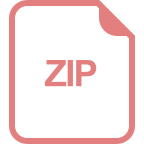
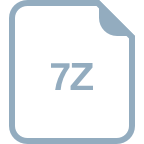
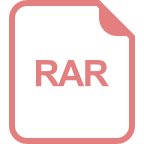
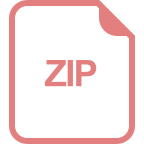
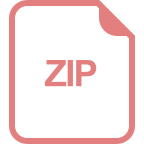
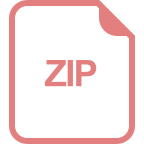
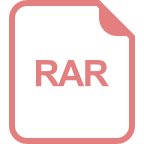
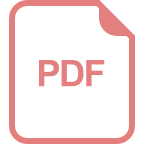
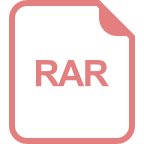
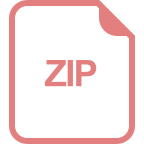
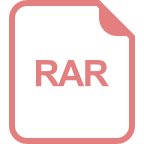
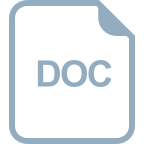
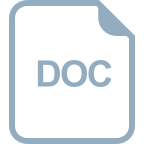
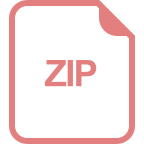
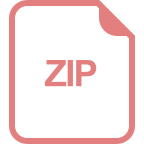