用C++语言实现一段代码,要求把一堆数重新排位,奇数在左边,偶数在右边
时间: 2024-10-28 20:09:34 浏览: 25
在C++中,你可以使用STL中的algorithm和partition函数来实现这个功能。这里有一个简单的例子,它将输入数组划分为两部分:左侧是奇数,右侧是偶数:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
void separateNumbers(std::vector<int>& numbers) {
// 使用partition函数,它会将元素分为两部分:小于或等于pivot(这里是0)的元素放在前面,大于pivot的元素放在后面
auto pivot_it = std::partition(numbers.begin(), numbers.end(), [](int num){ return num % 2 != 0; });
// 现在pivot_it指向了数组中第一个偶数的下一个位置。我们需要反转奇数部分,然后合并两者
std::reverse(numbers.begin(), pivot_it);
if (pivot_it != numbers.end()) { // 如果有偶数部分
std::copy(pivot_it, numbers.end(), pivot_it + 1); // 将偶数部分移到奇数部分之后
}
}
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9};
separateNumbers(numbers);
for (const auto& num : numbers) {
std::cout << num << " ";
}
std::cout << std::endl;
return 0;
}
```
当你运行这段代码,输出将是:`1 3 5 7 9 2 4 6 8`。
阅读全文
相关推荐



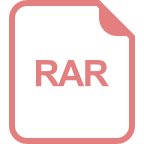

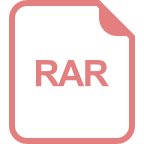






