搭建简易交互界面,通过界面按钮进行Windows<->Ubuntu协议收发验证
时间: 2024-10-24 11:09:46 浏览: 14
搭建简易交互界面通常涉及到编写客户端和服务端的应用程序,这里以Python语言为例,解释一下如何创建一个基本的Windows客户端(使用Tkinter库)和Ubuntu服务器(使用socket编程)来进行简单协议的收发验证。
**Windows客户端(Tkinter)**:
```python
import tkinter as tk
import socket
def send_data(text):
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
s.connect(('localhost', 12345)) # 假设Ubuntu服务器监听本地的12345端口
s.sendall(text.encode())
print("Data sent to Ubuntu.")
except Exception as e:
print(f"Error: {e}")
finally:
s.close()
button = tk.Button(text="Send", command=lambda: send_data(input("Enter data: ")))
root = tk.Tk()
button.pack()
root.mainloop()
```
**Ubuntu服务器(Python socket)**:
```python
import socket
from Crypto import Random # 加密模块(示例仅用于展示验证)
def handle_client(client_socket):
random_generator = Random.new().read
shared_key = b'some_secret_key' # 共享秘钥,实际项目应使用安全的随机生成
client_key = client_socket.recv(1024).strip() # 接收客户端发送的数据(这里假设是加密过的验证信息)
if verify_keys(shared_key, client_key): # 验证函数
print("Validation successful.")
# 进行进一步的交互或处理请求...
else:
print("Invalid key.")
# ...其他部分忽略...
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind(('localhost', 12345))
server_socket.listen(5)
while True:
client_socket, addr = server_socket.accept()
handle_client(client_socket)
```
在这个例子中,客户端点击按钮后会向服务器发送一条输入的消息。服务器接收消息后会对发送的信息进行验证(此处只做简化演示)。实际应用中需要考虑更安全的通信方式,比如加密。
阅读全文
相关推荐
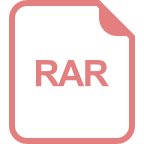
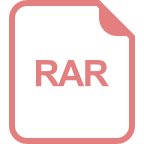
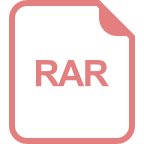
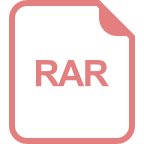
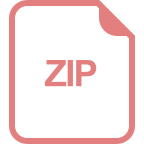
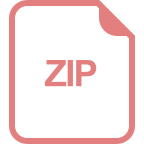
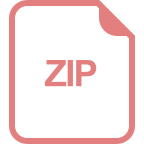
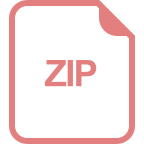
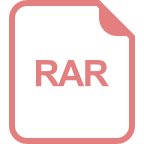
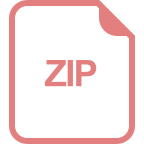
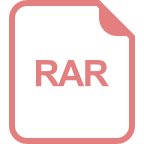
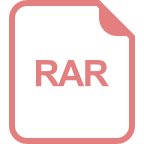
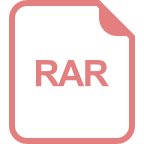
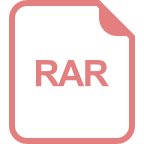
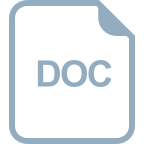
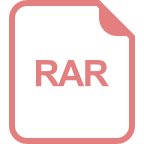
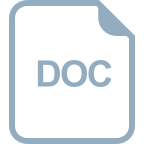
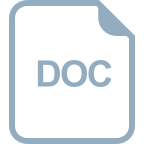
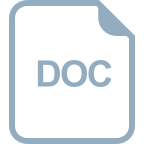