java ftpClient读取文件夹下全部文件并压缩zip
时间: 2024-10-21 20:17:44 浏览: 54
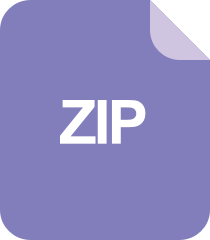
java FTP服务器文件上传下载,创建多级文件夹.zip
在Java中,你可以使用`java.util.zip`包中的`ZipOutputStream`类配合`FTPClient`来自Apache Commons Net库来读取FTP服务器上文件夹下的所有文件,并将它们压缩成ZIP文件。以下是一个简化的步骤:
1. **添加依赖**:
首先,确保已经添加了Apache Commons Net的FTP客户端依赖。如果你使用的是Maven,可以在pom.xml中添加:
```xml
<dependency>
<groupId>commons-net</groupId>
<artifactId>commons-net</artifactId>
<version>3.6</version>
</dependency>
```
2. **创建FTP连接**:
使用`FTPClient`类创建FTP连接并登录到服务器:
```java
FTPClient ftp = new FTPClient();
try {
ftp.connect("ftp.example.com");
ftp.login("username", "password");
} catch (Exception e) {
// 处理异常
}
```
3. **获取目录内容**:
利用`listFiles()`方法获取指定目录下的文件列表:
```java
String remoteDir = "/path/to/directory";
FileEntry[] entries = ftp.listFiles(remoteDir);
```
4. **创建ZIP输出流**:
初始化`ZipOutputStream`:
```java
FileOutputStream fos = new FileOutputStream("output.zip");
ZipOutputStream zos = new ZipOutputStream(fos);
```
5. **遍历文件并添加到ZIP**:
对于每个文件,构造`FileEntry`对象并写入到ZIP:
```java
for (FileEntry entry : entries) {
if (entry.isDirectory()) continue; // 如果是目录跳过
InputStream fis = ftp.retrieveFileStream(entry.getName());
ZipEntry zipEntry = new ZipEntry(entry.getName());
zos.putNextEntry(zipEntry);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
zos.write(buffer, 0, len);
}
zos.closeEntry();
fis.close();
}
```
6. **关闭资源**:
最后记得关闭所有已打开的流和连接:
```java
zos.close();
fos.close();
ftp.logout();
ftp.disconnect();
```
```
阅读全文
相关推荐
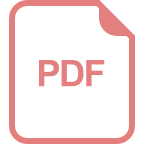
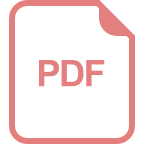


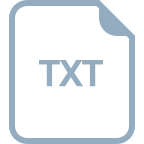
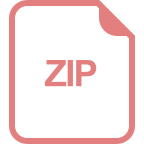
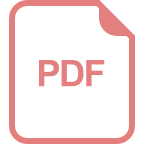
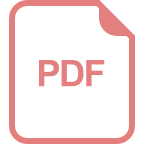
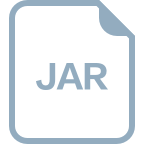
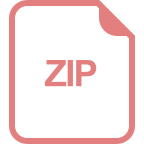
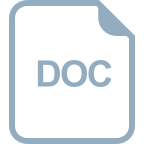
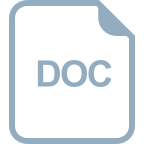
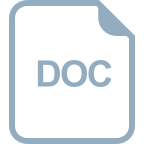


