Java 创建空MultipartFile文件
时间: 2023-08-15 17:09:58 浏览: 241
Java中的MultipartFile是一个接口,用于处理HTTP请求中的文件上传。它提供了一些方法来获取文件名、文件类型、文件大小等信息,并且可以通过getInputStream()方法获取文件的输入流,从而进行文件的读取和处理。在Spring框架中,MultipartFile常用于处理文件上传请求。
相关问题
java 解压缩MultipartFile 文件
在Java中,MultipartFile是Spring Framework中用于处理文件上传的接口。它提供了一些方法来操作上传的文件。然而,MultipartFile并没有直接提供解压缩文件的方法。
要解压缩MultipartFile文件,你需要将其转换为普通的Java IO文件类型,然后使用解压缩库来处理文件解压缩的操作。在你的引用中,你提到了一个私有方法 `unzip(MultipartFile file)` ,它使用MultipartFile文件进行解压缩操作。我假设这个方法中的逻辑是正确的。
为了实现解压缩MultipartFile文件的操作,你需要完成以下步骤:
1. 将MultipartFile文件转换为普通的Java IO文件类型。这可以通过创建一个临时文件并将MultipartFile的内容写入该文件来实现。你可以使用`file.transferTo()`方法将MultipartFile的内容写入Java IO文件。
2. 调用`unzip()`方法,将Java IO文件作为参数进行解压缩操作。该方法应该返回解压后的文件。
3. 将解压后的文件再次转换为MultipartFile类型,以便进一步处理或上传。
但是,请注意,将解压后的文件转换回MultipartFile类型并不是一个标准的操作。因为MultipartFile是Spring Framework中特定于文件上传的接口,它通常与文件上传相关的逻辑紧密结合。所以,如果你需要将解压后的文件再次转换为MultipartFile类型,你可能需要自定义一些逻辑来创建一个包含解压后文件内容的MultipartFile对象。
综上所述,要在Java中解压缩MultipartFile文件,你需要将MultipartFile转换为Java IO文件,然后使用解压缩库对该文件进行解压缩操作。如果需要将解压后的文件再次转换为MultipartFile类型,你可能需要自定义一些逻辑来创建相应的MultipartFile对象。
java如何创建MultipartFile对象
在Java中,`MultipartFile`是Spring MVC框架中的一个核心对象,用于处理文件上传。当你需要接收前端上传的文件并存储在服务器时,会用到这个类。以下是如何创建`MultipartFile`对象的步骤:
1. **使用表单提交**:
如果你使用HTML表单和`multipart/form-data`编码类型提交文件,你可以从`HttpServletRequest`中获取`MultipartFile`。例如,当用户上传文件后,控制器方法可能这样处理:
```java
@PostMapping("/upload")
public String handleFileUpload(@RequestParam("file") MultipartFile file) {
// 检查文件是否有效
if (file.isEmpty()) {
return "error";
}
// 保存文件到服务器
try {
saveFileToServer(file);
} catch (Exception e) {
return "error";
}
return "success";
}
```
2. **`saveFileToServer()`方法**:
这个方法负责处理文件的保存,通常会检查文件大小、类型,并将其写入磁盘或数据库:
```java
private void saveFileToServer(MultipartFile file) throws IOException {
String filename = Paths.get(file.getOriginalFilename()).getFileName().toString();
File destinationFile = new File("path/to/save/" + filename); // 替换为实际的保存路径
if (!destinationFile.exists()) {
destinationFile.createNewFile();
}
file.transferTo(destinationFile);
}
```
如果你使用的是其他上传方式(如`Servlet API`),则需要处理上传请求的`HttpServletRequest`对象,同样能获取`MultipartFile`。
阅读全文
相关推荐
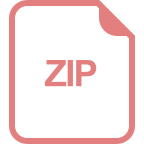













