C++ string 查找是否存在某个字符
时间: 2024-10-21 21:08:14 浏览: 27
在C++中,你可以使用 `find` 函数来查找字符串中是否包含特定字符。`std::string` 类提供了一个成员函数 `find`,它接受一个字符作为参数,并返回该字符首次出现在字符串中的位置(索引)。如果字符不存在,`find` 返回 `npos`(表示字符串结束的位置,即 `size_t(-1)`)。
以下是示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
char search_char = 'o';
size_t index = str.find(search_char);
if (index != std::string::npos) {
std::cout << "Character '" << search_char << "' is present at position " << index << std::endl;
} else {
std::cout << "Character '" << search_char << "' is not found in the string." << std::endl;
}
return 0;
}
```
在这个例子中,如果找到了字符 `'o'`,程序会输出它的位置;如果没有找到,就会输出提示信息。
相关问题
c++字符串包含某个字符
在C语言中,可以使用strstr函数来在字符串中查找另一个字符串。该函数的定义为char * strstr(const char *str1, const char *str2)。
在C++中,可以使用string类的find函数来查找字符串是否存在。该函数的功能是在string对象中查找参数string类型的字符串是否存在,如果存在,则返回其起始位置;如果不存在,则返回string::npos。
下面是一个示例代码,展示了如何使用find函数来判断字符串是否包含某个字符:
#include <iostream>
#include <string>
using namespace std;
int main() {
string a = "abcdefghigklmn";
string b = "def";
string c = "123";
string::size_type idx;
idx = a.find(b); //在a中查找b
if (idx == string::npos) //不存在
cout << "not found" << endl;
else //存在
cout << "found" << endl;
idx = a.find(c); //在a中查找c
if (idx == string::npos) //不存在
cout << "not found" << endl;
else //存在
cout << "found" << endl;
return 0;
}
在上述代码中,a是目标字符串,b是要查找的字符串,c是另一个要查找的字符串。通过调用find函数并判断返回值是否等于string::npos,可以判断字符串是否包含某个字符。如果返回值等于string::npos,则表示字符串不存在;否则,表示字符串存在。
c++从一个字符串中查找某个字符串并删除的代码
以下是一个 Python 代码示例,可以从一个字符串中查找某个字符串并删除:
```
string = "Hello, world!"
substring = "world"
new_string = string.replace(substring, "")
print(new_string)
```
这段代码将在字符串 `string` 中查找子字符串 `substring` 并将其删除,最后输出新的字符串 `new_string`。
阅读全文
相关推荐
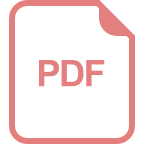
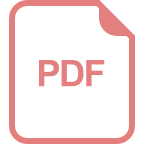
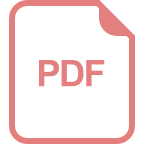
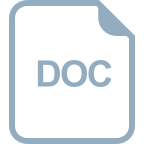
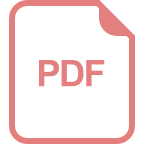
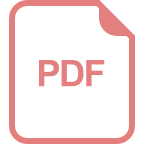
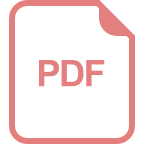
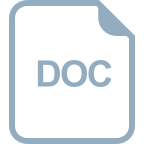
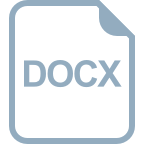
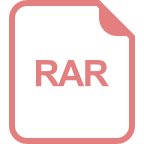
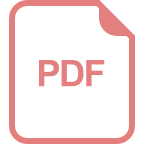
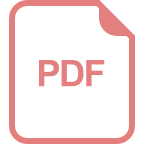
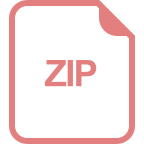
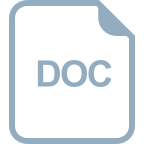
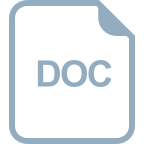