cpphttplib 文件流式上传服务端和客户端示例
时间: 2024-11-03 21:18:23 浏览: 26
当然可以!cpphttplib是一个C++库,用于构建HTTP服务器和客户端。下面是一个使用cpphttplib的文件流式上传服务端和客户端示例的简单介绍:
文件流式上传服务端示例:
```cpp
#include <iostream>
#include <fstream>
#include <cpphttplib/cpphttplib.h>
using namespace std;
void uploadFile(string filePath) {
// 创建HTTP服务器
auto server = make_shared<http::server::http_server>();
// 设置服务器端口
server->listen(8888);
// 处理文件上传请求的路由
server->on(request(methods::POST), "/upload", [=](http::server::request_ptr req, http::server::response_ptr res) {
// 获取上传的文件路径
auto filePath = req->header("Content-Disposition")->value().as<string>();
auto file = req->body().as<string>();
// 将文件保存到本地磁盘
ofstream outfile(filePath, ios::binary);
outfile << file;
outfile.close();
// 返回响应
res->send_response(200);
});
// 启动服务器
server->run();
}
int main() {
uploadFile("path/to/your/file"); // 将文件路径替换为你要上传的实际文件路径
return 0;
}
```
上述代码创建了一个HTTP服务器,监听端口8888。当收到POST请求时,它会解析请求体中的文件内容,并将其保存到指定的文件路径。最后,服务器返回一个200的响应表示成功。你可以将实际文件路径替换为你要上传的文件路径。
文件流式上传客户端示例:
```cpp
#include <iostream>
#include <fstream>
#include <cpphttplib/cpphttplib.h>
#include <vector>
using namespace std;
using namespace cpphttplib;
void uploadFile(string filePath) {
// 发送POST请求进行文件上传
auto client = make_shared<http::client>();
auto request = client->request(methods::POST);
request->add_header("Content-Type", "multipart/form-data"); // 设置正确的Content-Type头标
request->set_target("localhost", 8888, "/upload"); // 设置目标服务器的地址和端口以及路由路径
request->body()->write("filename=" + filePath); // 将文件名添加到请求体中,用于保存文件路径到本地磁盘
request->body()->write("\r\n"); // 添加换行符,确保请求体正确格式化
request->body()->write("filecontent=" + string(filePath)); // 将文件内容写入请求体中,以便服务器保存文件内容到本地磁盘
request->body()->flush(); // 确保所有内容都已写入请求体中,以便服务器正确解析请求体中的内容
auto response = client->post(request); // 发送请求并获取响应
if (response->status() == 200) { // 检查响应状态码是否为200表示成功上传文件
cout << "File uploaded successfully." << endl; // 输出成功消息
} else {
cout << "Failed to upload file." << endl; // 输出失败消息或错误信息
}
}
int main() {
uploadFile("path/to/your/file"); // 将文件路径替换为你要上传的实际文件路径,例如"path/to/your/file.txt"
return 0;
}
```
上述代码使用cpphttplib库发送一个POST请求进行文件上传。在请求体中,它添加了正确的Content-Type头标,并将文件名和文件内容写入请求体中。最后,它检查响应状态码是否为200,以确定文件是否成功上传。你可以将实际文件路径替换为你要上传的文件路径。
阅读全文
相关推荐
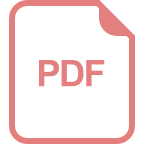
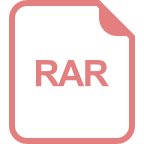
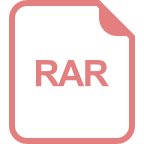
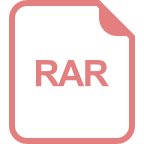
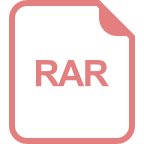
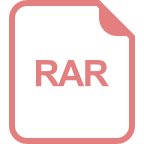
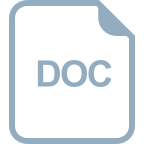
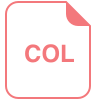
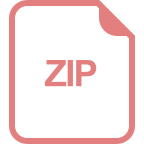
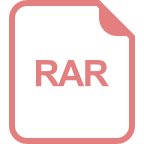
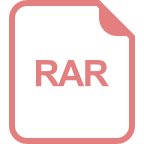
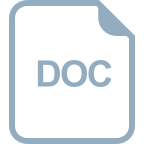
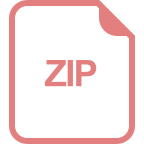
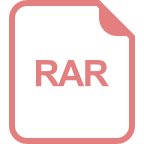
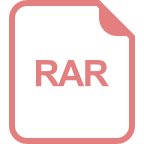
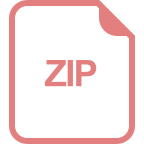
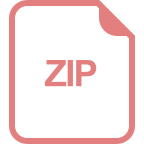
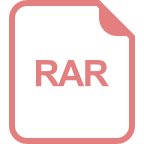
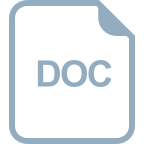