在Android Studio中用ImageSitcher实现微信大图轮播功能,包括动画效果的全部代码
时间: 2024-09-30 14:05:27 浏览: 37
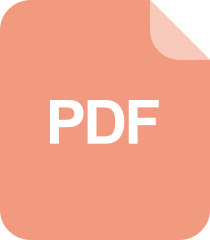
Android实现图片轮播效果的两种方法

在Android Studio中,使用`ImageSwitcher`实现微信风格的大图轮播功能通常需要几个步骤。首先,你需要在布局文件中添加`ImageSwitcher`组件,并设置一些基本属性如宽度、高度以及背景颜色。然后,你需要创建一个包含图片路径的数组,用于存储轮播图内容。
下面是一个简单的示例代码:
```xml
<!-- layout/activity_main.xml -->
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<ImageView
android:id="@+id/image_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:scaleType="centerCrop" />
<!-- ImageSwitcher -->
<ImageView
android:id="@+id/image_switcher"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:visibility="gone" />
</LinearLayout>
```
接着,在对应的Activity Java或Kotlin代码中,你可以这样做:
```java
// MainActivity.java (Java)
import android.content.res.Resources;
import android.graphics.drawable.Drawable;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.ImageSwitcher;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity {
private ImageSwitcher imageSwitcher;
private String[] imagePaths = { "path_to_image1", "path_to_image2", ... }; // 图片URLs
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imageSwitcher = findViewById(R.id.image_switcher);
imageSwitcher.setImageResource(R.drawable.default_placeholder); // 设置初始图片
imageSwitcher.setFactory(new ViewFactory() {
@Override
public View makeView() {
ImageView imageView = new ImageView(MainActivity.this);
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
return imageView;
}
});
ArrayAdapter<String> adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, imagePaths);
imageSwitcher.setAutoStart(true); // 开启自动轮播
imageSwitcher.setInAnimation(getResources().getDrawable(android.R.anim.fade_in));
imageSwitcher.setOutAnimation(getResources().getDrawable(android.R.anim.fade_out));
imageSwitcher.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
imageSwitcher.setImageDrawable(getDrawable(imagePaths[position]));
}
@Override
public void onNothingSelected(AdapterView<?> parent) {
// Do nothing
}
});
}
private Drawable getDrawable(String path) {
Resources resources = getResources();
try {
return resources.getDrawable(Uri.parse(path), null);
} catch (Resources.NotFoundException e) {
return resources.getDrawable(R.drawable.default_placeholder);
}
}
}
```
在这个例子中,我们设置了轮播开始时的默认图片,设置了滑动动画效果,并监听了选中的图片改变事件。记得将`imagePaths`数组替换为实际的图片路径,以及处理可能出现的资源找不到的情况。
阅读全文
相关推荐
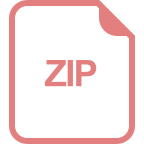
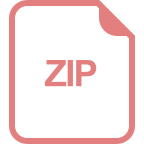














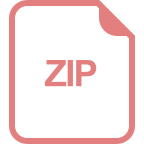
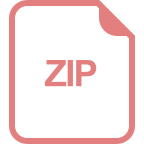