如何在C#中实现三层架构,并使用ORM技术来简化数据库操作?请结合泛型集合提供实现细节。
时间: 2024-11-06 18:33:57 浏览: 29
在C#中实现三层架构的过程中,使用ORM技术可以显著提高开发效率并降低数据库操作的复杂性。以下是详细的实现步骤和关键点:
参考资源链接:[C#三层架构详解:从设计到实现](https://wenku.csdn.net/doc/57bv23ecrm?spm=1055.2569.3001.10343)
1. **数据访问层(DAL)**:首先,你需要设计数据访问层,这通常涉及到定义接口和实现类来封装数据库操作。使用ORM框架如Entity Framework时,你可以利用其提供的上下文(Context)对象来与数据库交互。例如,创建一个继承自`DbContext`的类来表示数据模型,并在其中定义数据集(DbSet)。
```csharp
public class MyDbContext : DbContext
{
public DbSet<MyEntity> MyEntities { get; set; }
// 其他DbSet定义
}
```
2. **业务逻辑层(BLL)**:在业务逻辑层中,定义业务方法来处理业务逻辑。这些方法不应该直接引用数据库操作,而是通过数据访问层提供的接口或类来获取所需数据。这样可以保证业务逻辑层的独立性和可测试性。
```csharp
public class MyBusinessLogic
{
private IMyEntityRepository _repository;
public MyBusinessLogic(IMyEntityRepository repository)
{
_repository = repository;
}
public List<MyEntity> GetAllEntities()
{
return _repository.GetAll().ToList();
}
}
```
3. **表示层**:表示层通过调用业务逻辑层的方法来处理用户请求,并将结果展示给用户。在这个层中,你可能会用到如MVC或WebAPI等框架来创建用户界面和服务接口。
```csharp
public class MyController : Controller
{
private MyBusinessLogic _businessLogic;
public MyController(MyBusinessLogic businessLogic)
{
_businessLogic = businessLogic;
}
public ActionResult GetEntities()
{
var entities = _businessLogic.GetAllEntities();
return View(entities);
}
}
```
4. **泛型集合**:泛型集合在三层架构中扮演着重要角色,特别是在数据访问层。使用泛型集合可以提高代码的复用性和类型安全。例如,在数据访问层,你可以定义泛型接口和实现类来处理数据集合。
```csharp
public interface IRepository<TEntity> where TEntity : class
{
IEnumerable<TEntity> GetAll();
// 其他CRUD操作
}
public class EntityRepository<TEntity> : IRepository<TEntity> where TEntity : class
{
public MyDbContext _context;
public EntityRepository(MyDbContext context)
{
_context = context;
}
public IEnumerable<TEntity> GetAll()
{
return _context.Set<TEntity>().ToList();
}
}
```
通过上述步骤,你可以在C#中实现三层架构,并有效地利用ORM技术来简化数据库操作。这种架构模式不仅有助于代码的组织,还能提高软件的可维护性和可扩展性。如果想要深入学习三层架构的细节和更多实现技巧,推荐阅读《C#三层架构详解:从设计到实现》。这本书提供了详细的理论知识和实例分析,能够帮助你更好地理解和掌握C#三层架构的设计和实现。
参考资源链接:[C#三层架构详解:从设计到实现](https://wenku.csdn.net/doc/57bv23ecrm?spm=1055.2569.3001.10343)
阅读全文
相关推荐
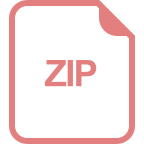
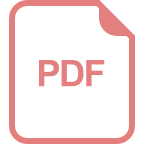
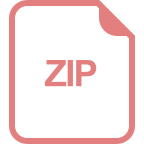


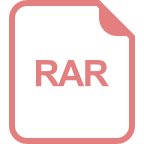
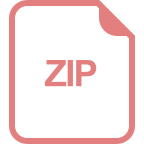
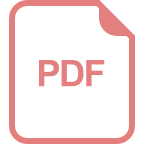
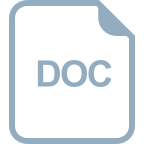
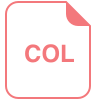
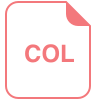
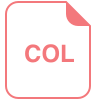
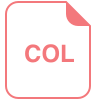
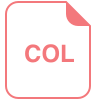
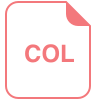
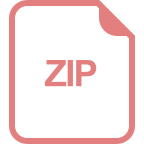