用C++基础的数组方法写
时间: 2024-11-30 16:14:09 浏览: 12
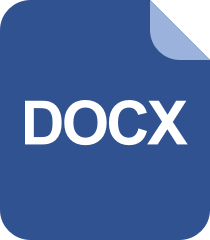
C++指针 数组 内存释放.docx

在C++中,我们可以使用基本的数组数据结构来实现寻找重复数字的功能。以下是一个简单的示例:
```cpp
#include <iostream>
#include <vector>
std::string findDuplicates(int* arr, int n) {
std::vector<int> numSet; // 使用vector代替set,因为set在C++标准库中没有内置
for (int i = 0; i < n; ++i) {
if (std::find(numSet.begin(), numSet.end(), arr[i]) != numSet.end()) { // 检查元素是否已经存在于vector中
numSet.push_back(arr[i]); // 添加到vector中
} else {
numSet.clear(); // 如果当前元素未找到,清空vector以便继续搜索
}
}
if (!numSet.empty()) { // 如果vector非空,有重复数字
std::stringstream ss;
for (int num : numSet) {
ss << num << " ";
}
return ss.str();
} else {
return "Not Found";
}
}
int main() {
int n;
std::cout << "Enter the number of cards: ";
std::cin >> n;
int* numbers = new int[n]; // 创建动态数组
std::cout << "Enter the numbers: ";
for (int i = 0; i < n; ++i) {
std::cin >> numbers[i];
}
std::string result = findDuplicates(numbers, n);
delete[] numbers; // 释放内存
std::cout << result << std::endl;
return 0;
}
```
在这个例子中,我们首先使用`std::vector`存储遇到过的数字。然后遍历数组,如果某个数字已经在`numSet`中,就把它添加到结果中。如果没找到,就清空`numSet`继续搜索。最后,根据`numSet`是否为空返回相应的消息。
**相关问题--:**
1. C++标准库中为什么不用`set`而用`vector`?
2. 动态分配数组`numbers`的原因是什么?
3. 如何改进这段代码使其更健壮,处理用户输入错误的情况?
阅读全文
相关推荐
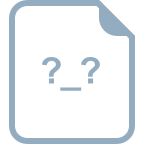
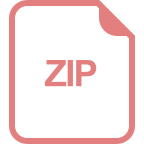
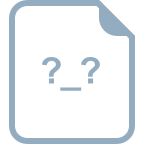
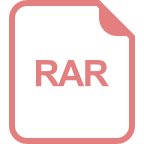
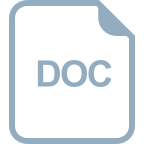
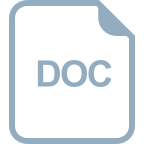
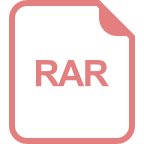
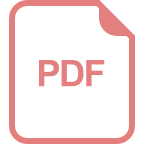
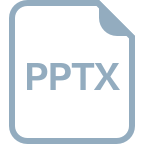
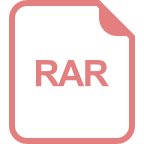
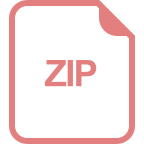
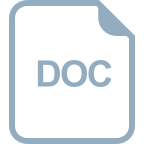
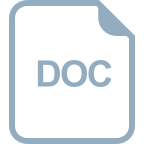
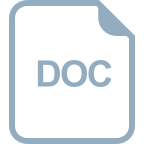
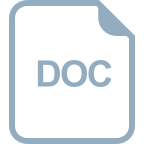
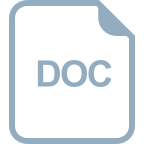
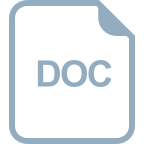
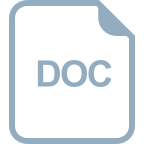