用C语言写一个项目,可以查找具体年份和具体月份的公历
时间: 2024-12-24 18:42:08 浏览: 4
在C语言中创建一个简单的项目来查找特定年份和月份的公历,你可以编写一个程序,它接受用户输入的年份和月份,然后使用`<time.h>`库中的`mktime()`函数和`strftime()`函数来处理。以下是一个基本示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 函数来检查给定年份是否为闰年
int is_leap_year(int year) {
if (year % 4 != 0)
return 0;
else if (year % 100 != 0)
return 1;
else if (year % 400 != 0)
return 0;
else
return 1;
}
void print_calendar(int year, int month) {
struct tm date = {0};
// 设置默认的时间,我们将只关注月份和年份
date.tm_mon = month - 1; // 月份从0开始计数
date.tm_year = year - 1900; // 使用格里高利历
// 将tm结构体转换为时间戳,然后再次转换为字符串格式
time_t timestamp = mktime(&date);
char calendar[80];
strftime(calendar, sizeof(calendar), "%B %Y", &date);
printf("Calendar for %s:\n", calendar);
// 这里你可以添加一行或多行代码来显示每个月的具体日期,例如使用循环和printf()
// 由于这涉及到实际的日期计算,这里简化为打印"1 to 31"
for (int day = 1; day <= 31; day++) {
printf("%d ", day);
if (day == 31 || (day > 28 && is_leap_year(year))) { // 结束线
printf("\n");
}
}
}
int main() {
int year, month;
printf("Enter the year and month (YYYY MM): ");
scanf("%d %d", &year, &month);
if (month >= 1 && month <= 12) {
print_calendar(year, month);
} else {
printf("Invalid month!\n");
}
return 0;
}
```
这个程序首先检查输入的月份是否有效,然后创建一个表示指定月份的`tm`结构体,并将其转换为可读的日期格式。注意,这个示例没有详细列出每一天的详细日历,仅展示了月份的基本框架和闰年的判断。
阅读全文
相关推荐
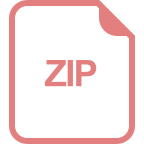
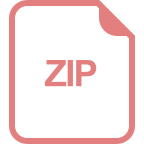
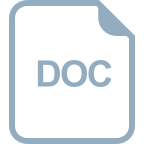

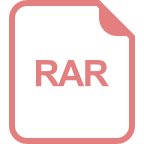
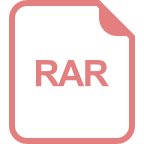
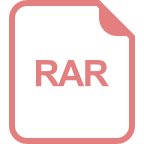
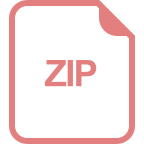
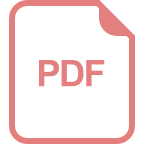
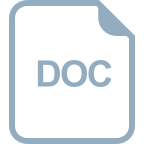
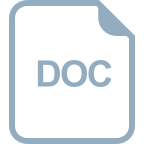
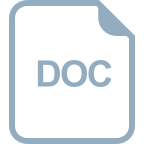
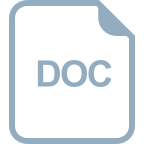
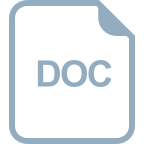
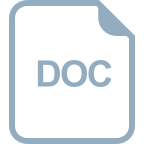
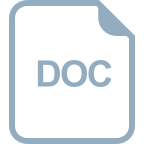
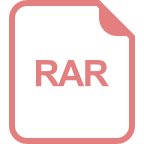
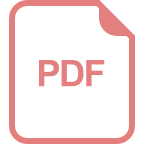