mqtt 解析包代码
时间: 2023-09-08 13:01:55 浏览: 89
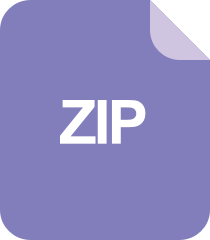
php mqtt代码,实现通信
MQTT(Message Queuing Telemetry Transport)是一种轻量级的通讯协议,常用于物联网设备之间的通信。下面是一个简单的MQTT解析包的代码示例。
```python
import struct
def parse_mqtt_packet(packet):
# 解析固定报头的第一个字节,获取报文类型和标志位
packet_type = packet[0] >> 4
flags = packet[0] & 0x0F
print("Packet Type:", packet_type)
print("Flags:", flags)
# 解析剩余长度字段
remaining_length = 0
multiplier = 1
index = 1
while True:
digit = packet[index]
remaining_length += (digit & 127) * multiplier
multiplier *= 128
index += 1
if (digit & 128) == 0:
break
print("Remaining Length:", remaining_length)
# 解析可变报头和负载数据
if packet_type == 1:
# 连接请求报文
protocol_name_length = struct.unpack("!H", packet[index:index+2])[0]
index += 2
protocol_name = packet[index:index+protocol_name_length].decode("utf-8")
index += protocol_name_length
print("Protocol Name:", protocol_name)
protocol_version = packet[index]
index += 1
print("Protocol Version:", protocol_version)
flags = packet[index]
index += 1
print("Flags:", flags)
keep_alive = struct.unpack("!H", packet[index:index+2])[0]
index += 2
print("Keep Alive:", keep_alive)
client_id_length = struct.unpack("!H", packet[index:index+2])[0]
index += 2
client_id = packet[index:index+client_id_length].decode("utf-8")
index += client_id_length
print("Client ID:", client_id)
elif packet_type == 3:
# 发布报文
topic_length = struct.unpack("!H", packet[index:index+2])[0]
index += 2
topic = packet[index:index+topic_length].decode("utf-8")
index += topic_length
print("Topic:", topic)
message = packet[index:]
print("Message:", message)
# 解析完整个报文后,可以根据需要进行其他操作,如校验报文合法性、执行相应的逻辑等。
# 测试示例
packet = b"\x10\xCC\x00\x04\x4D\x51\x54\x54\x04\x02\x00\x0A\x4D\x79\x43\x6C\x69\x65\x6E\x74\x49\x44"
parse_mqtt_packet(packet)
```
以上代码是一个简单的MQTT解析包示例,用于解析MQTT连接请求和发布报文。函数`parse_mqtt_packet`接收一个字节数组作为参数,通过解析字节数组中的字段,可以获取报文类型、标志位、剩余长度、可变报头和负载数据等信息。可以根据业务需要,进一步扩展代码以支持其他MQTT报文类型的解析。
阅读全文
相关推荐
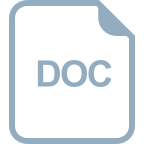
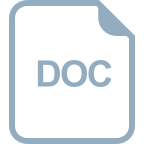
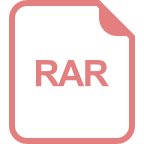
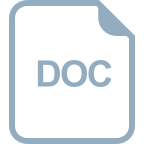
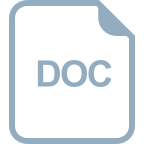



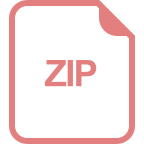
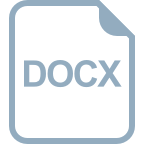
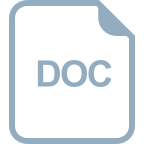
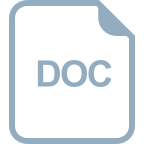
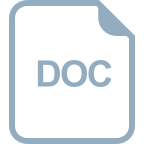
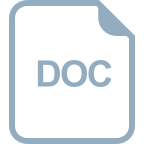
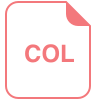

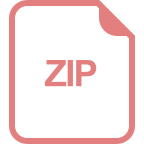
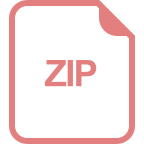