python pil批量读取图片
时间: 2024-09-18 21:00:58 浏览: 21
在Python中,PIL(Python Imaging Library,现在更常用的是Pillow库)是一个用于处理图像的强大库。如果你想批量读取图片,可以按照以下步骤操作:
1. 首先,你需要安装Pillow库,如果还没有安装,可以使用pip进行安装:
```
pip install pillow
```
2. 然后,创建一个函数来读取单张图片:
```python
from PIL import Image
def load_image(file_path):
with Image.open(file_path) as img:
return img
```
3. 使用os模块遍历指定目录下的所有图片文件,并逐个读取:
```python
import os
image_dir = 'path/to/image/directory'
images = [load_image(os.path.join(image_dir, filename)) for filename in os.listdir(image_dir) if filename.endswith('.jpg') or filename.endswith('.png')]
```
这里假设你只对`.jpg`和`.png`类型的图片感兴趣。
4. `images`列表现在包含了目录下所有符合条件的图片对象。
相关问题
python 批量读取图片PIL
在Python中,使用Pillow库(PIL的分支)批量读取图片通常涉及遍历文件夹并逐张打开图片。以下是一个简单的示例,展示如何使用PIL的`Image`模块批量读取目录下的所有图片:
```python
from PIL import Image
import os
def batch_read_images(directory):
images = []
for filename in os.listdir(directory): # 遍历指定目录
if filename.endswith(('.jpg', '.png', '.jpeg')): # 检查文件是否为图片
try:
img = Image.open(os.path.join(directory, filename)) # 打开图片
images.append(img) # 将图片添加到列表
except Exception as e:
print(f"无法打开文件 {filename}: {e}")
return images
directory_path = "your_image_directory" # 替换为你需要读取图片的路径
images_list = batch_read_images(directory_path)
```
在这个例子中,我们首先导入了所需的模块,然后定义了一个函数`batch_read_images`,它接受一个目录作为输入。通过`os.listdir`获取目录中的所有文件名,然后判断每个文件是否是图片(这里是通过检查文件扩展名)。如果是图片,就尝试使用`Image.open`打开,如果失败则打印错误信息。
python批量读取图片
你可以使用Python的Pillow库来批量读取图片。下面是一个简单的代码示例,可以将指定文件夹中的所有图片读取到一个列表中:
```python
from PIL import Image
import os
# 指定图片所在文件夹路径
folder_path = "/path/to/folder/"
# 读取文件夹中的所有文件
file_list = os.listdir(folder_path)
# 创建空列表用于存储图片
image_list = []
# 遍历文件列表
for file_name in file_list:
# 判断文件是否为图片
if file_name.endswith(".jpg") or file_name.endswith(".png"):
# 拼接文件路径
file_path = os.path.join(folder_path, file_name)
# 打开图片并添加到列表中
image = Image.open(file_path)
image_list.append(image)
```
这个示例假设你的图片文件全部存储在同一个文件夹中,你需要将 `folder_path` 变量替换为你实际的文件夹路径。另外,你也可以根据你的需求修改代码以适应不同的图片格式和文件夹结构。
相关推荐
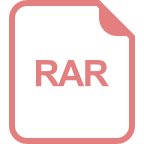
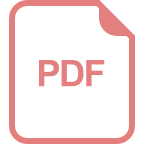
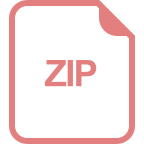












