用标签显示一道简单的测试题,答案使用单击按钮列出用户选择答案按后在另一个标签中显示结果或说明。Java代码
时间: 2024-10-28 08:07:08 浏览: 23
在Java中,你可以使用Swing库来创建这样的简单GUI应用。下面是一个基本的示例,展示了如何使用JFrame、JLabel、JButton以及JOptionPane来实现这个功能:
```java
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class QuizApp {
private JFrame frame;
private JLabel questionLabel;
private JButton answerButton;
private String[] answers = {"A", "B", "C", "D"}; // 假设题目有四个选项
private int correctAnswerIndex = 0; // 标记正确答案位置
public static void main(String[] args) {
EventQueue.invokeLater(() -> {
try {
QuizApp window = new QuizApp();
window.frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
});
}
public QuizApp() {
initialize();
}
private void initialize() {
frame = new JFrame("Quiz App");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 创建问题标签
questionLabel = new JLabel("这是你的问题...");
frame.add(questionLabel);
// 创建答案按钮
answerButton = new JButton("查看答案");
answerButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int selectedAnswer = JOptionPane.showOptionDialog(frame,
"请选择答案:" + answers[correctAnswerIndex],
"选择",
JOptionPane.YES_NO_OPTION,
JOptionPane.PLAIN_MESSAGE,
null,
answers,
answers[correctAnswerIndex]);
if (selectedAnswer == answers[correctAnswerIndex]) {
JOptionPane.showMessageDialog(frame, "恭喜,你答对了!");
} else {
JOptionPane.showMessageDialog(frame, "很遗憾,答错了 :(");
}
}
});
frame.getContentPane().add(answerButton);
}
}
```
在这个例子中,当用户点击"查看答案"按钮时,会弹出一个带有四个选项的对话框让用户选择。然后程序检查用户的输入是否匹配预设的答案,给出相应的结果。
阅读全文
相关推荐

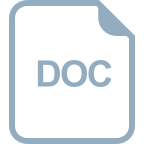


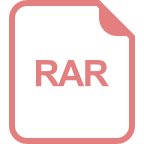












