map<int,int> v={{"1,2"},{"2,3"}}; for (map<int,int>::iterator it=v.begin();it!=v.end()){ cout<<*it->first<<endl; },这么写可以吗
时间: 2024-11-05 13:28:21 浏览: 19
这段代码尝试遍历一个包含字符串键值对的`map<int,int>`,但是按照C++的语法,直接使用`*it->first`可能会有问题,因为`first`通常指向的是`std::pair<const Key, T>`的键部分,而在这个例子中,键实际上是字符串,而不是整数。
正确的做法应该是先将字符串转换成整数再输出。这里假设你可以通过某种方式成功解析字符串并将其转换成`int`。以下是修改后的代码示例:
```cpp
#include <string>
using namespace std;
// 假设有一个函数convertToInt能将字符串转换成整数
int convertToInt(const string& str) {
// 实现字符串转整数的具体逻辑...
}
for (auto it = v.begin(); it != v.end(); ++it) {
cout << convertToInt(*it->first) << endl;
}
```
如果`convertToInt`不存在或者解析失败,你需要处理这种异常情况。另外,如果你确定键已经是整数形式,那么可以直接使用`it->first`,无需转换。
阅读全文
相关推荐
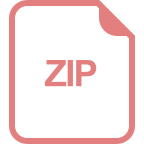