时空多图卷积神经网络的代码
时间: 2024-03-30 12:32:01 浏览: 141
时空多图卷积神经网络(ST-GCN)是一种用于处理时空数据的深度学习模型。它在图卷积神经网络(GCN)的基础上进行了扩展,可以有效地处理时序数据和空间关系。
以下是一个简单的时空多图卷积神经网络的代码示例:
```python
import torch
import torch.nn as nn
from torch_geometric.nn import ChebConv
class STGCN(nn.Module):
def __init__(self, num_nodes, in_channels, num_classes):
super(STGCN, self).__init__()
self.num_nodes = num_nodes
self.in_channels = in_channels
self.num_classes = num_classes
self.temporal_conv1 = ChebConv(in_channels, 64, K=2)
self.temporal_conv2 = ChebConv(64, 64, K=2)
self.spatial_conv1 = ChebConv(64, 64, K=2)
self.spatial_conv2 = ChebConv(64, num_classes, K=2)
def forward(self, x, edge_index):
# x: [batch_size, in_channels, num_nodes, num_timesteps]
# edge_index: [2, num_edges]
x = x.permute(0, 3, 1, 2) # [batch_size, num_timesteps, in_channels, num_nodes]
batch_size, num_timesteps, _, _ = x.size()
x = x.reshape(batch_size * num_timesteps, self.in_channels, self.num_nodes)
edge_index = edge_index.unsqueeze(0).repeat(batch_size * num_timesteps, 1, 1)
x = self.temporal_conv1(x, edge_index)
x = self.temporal_conv2(x, edge_index)
x = x.reshape(batch_size, num_timesteps, 64, self.num_nodes)
x = x.permute(0, 3, 2, 1) # [batch_size, num_nodes, 64, num_timesteps]
x = x.reshape(batch_size * self.num_nodes, 64, num_timesteps)
edge_index = edge_index.squeeze(0)
x = self.spatial_conv1(x, edge_index)
x = self.spatial_conv2(x, edge_index)
x = x.reshape(batch_size, self.num_nodes, self.num_classes, num_timesteps)
x = x.permute(0, 3, 2, 1) # [batch_size, num_timesteps, num_classes, num_nodes]
return x
```
这段代码实现了一个简单的ST-GCN模型,包括两个时间卷积层和两个空间卷积层。你可以根据自己的数据和需求进行修改和扩展。
阅读全文
相关推荐
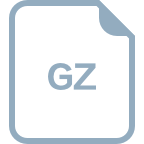
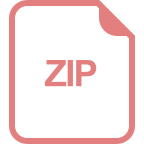
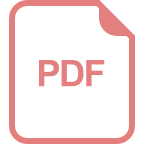
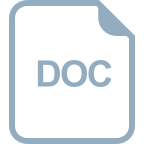
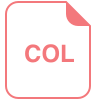
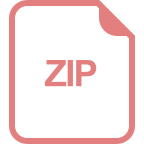
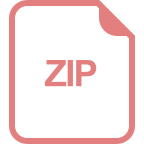
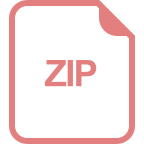
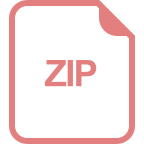
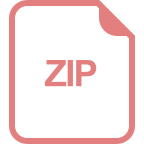
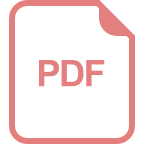
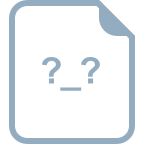
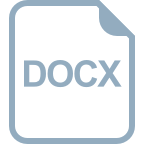
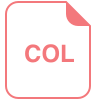
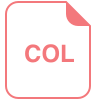
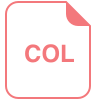
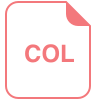
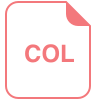