如何在Jupyter Notebook中创建一个自定义类并使用它来绘制圆和长方形?
时间: 2024-12-15 19:23:37 浏览: 10
在Jupyter Notebook中,你可以使用Python语言来创建自定义类并实例化它们来绘制图形。首先,你需要导入相关的绘图库如`matplotlib.pyplot`。以下是一个简单的例子,我们创建一个名为`Shape`的基类,并有两个子类`Circle`和`Rectangle`,分别用于绘制圆形和矩形:
```python
import matplotlib.pyplot as plt
from abc import ABC, abstractmethod
# 创建一个抽象基类Shape
class Shape(ABC):
@abstractmethod
def draw(self):
pass
# 子类Circle
class Circle(Shape):
def __init__(self, center=(0, 0), radius=1):
self.center = center
self.radius = radius
def draw(self):
plt.scatter(self.center[0], self.center[1], s=self.radius ** 2)
plt.gca().add_patch(plt.Circle(self.center, self.radius, fill=False))
plt.show()
# 子类Rectangle
class Rectangle(Shape):
def __init__(self, corner1=(0, 0), corner2=(1, 1)):
self.corner1 = corner1
self.corner2 = corner2
def draw(self):
x1, y1 = self.corner1
x2, y2 = self.corner2
plt.plot([x1, x2], [y1, y2])
plt.fill_between([x1, x2], [y1, y2], color='blue', alpha=0.5)
plt.show()
# 使用新创建的类
circle = Circle(center=(2, 3), radius=2)
circle.draw() # 绘制一个圆
rectangle = Rectangle(corner1=(-1, -1), corner2=(4, 5))
rectangle.draw() # 绘制一个矩形
```
在这个例子中,`draw()`方法是抽象方法,子类需要实现它来提供特定形状的绘制逻辑。
阅读全文
相关推荐
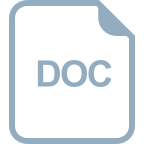
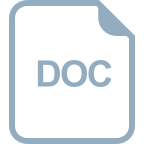
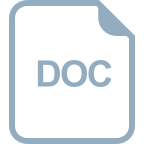
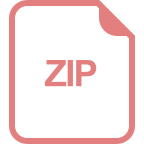
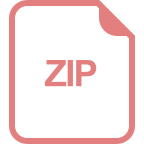
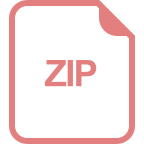
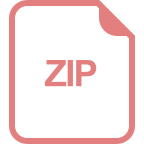
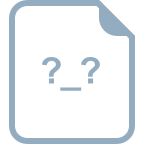
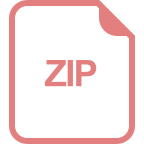
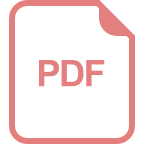
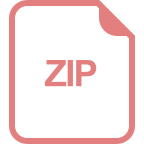
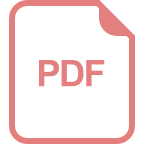
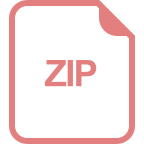
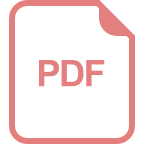
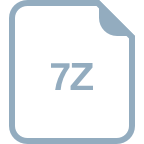
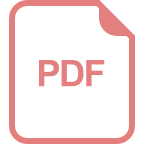
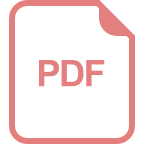