python爬虫某一股票数据
时间: 2024-12-26 22:26:35 浏览: 2
### 使用Python编写爬虫获取特定股票数据
#### 选择合适的工具和库
对于简单的静态网页,`Requests` 和 `BeautifulSoup` 是理想的选择。这两个库能够快速有效地解析HTML文档并提取所需的信息[^2]。
```python
import requests
from bs4 import BeautifulSoup
def get_stock_info(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 假设目标页面有一个ID为'stock-price'的标签包含了股价信息
price_tag = soup.find(id='stock-price')
stock_price = float(price_tag.string.strip())
return stock_price
```
然而,如果目标网站依赖JavaScript加载内容,则可能需要采用更强大的解决方案如 `Selenium` 来模拟真实用户的交互过程。
#### 利用专门的金融数据接口
为了简化操作并提高可靠性,还可以考虑使用专门为金融市场设计的数据服务API,比如Yahoo Finance API。借助第三方库 `yfinance` 可以方便地查询个股的历史行情和其他重要指标[^3]。
```python
import yfinance as yf
apple = yf.Ticker("AAPL")
# 获取苹果公司的股息分配记录
print(apple.dividends)
# 查询最近一年的日K线图
hist = apple.history(period="1y")
print(hist)
```
#### 安装必要的软件包
无论采取哪种方案,都需要先安装相应的Python模块。可以通过pip命令一次性完成多个包的下载与配置工作[^4]:
```bash
pip install requests beautifulsoup4 selenium yfinance pandas openpyxl
```
#### 数据保存至文件
当收集到足够的历史交易日志之后,通常会希望将其导出成易于阅读的形式以便后续分析。下面的例子展示了怎样把获得的结果存入Excel表格内[^5]:
```python
import pandas as pd
data_all = []
for url in urls:
try:
info = fetch_url_content(url)
clean_text = remove_spaces_with_replace(info['text'])
row = {
"Date": info["date"],
"Price": extract_price(clean_text),
...
}
data_all.append(row)
except Exception as e:
print(f"Error processing {url}: ", str(e))
df = pd.DataFrame(data_all)
writer = pd.ExcelWriter('output.xlsx', engine='openpyxl')
df.to_excel(writer, index=False)
writer.save()
```
阅读全文
相关推荐
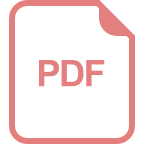
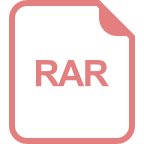





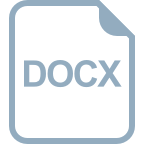
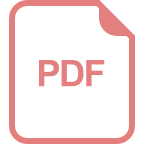
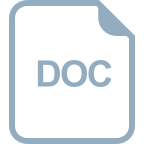
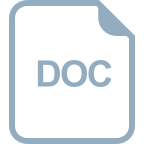
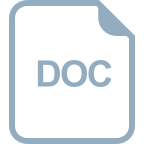
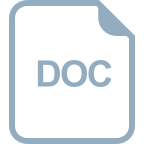
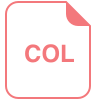
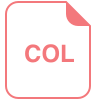
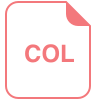
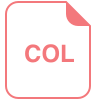
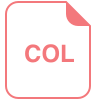
