用java写一个能改变类中属性名输出的自定义注解,不加注解默认原属性名,全部打印输出
时间: 2024-04-11 19:33:48 浏览: 265
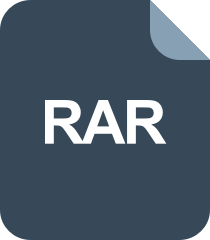
java自定义注解
以下是一个示例代码,实现了一个自定义注解 `@JsonProperty`,用于改变类中属性名的输出。如果没有使用 `@JsonProperty` 注解,则默认输出原属性名。
```java
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
import java.lang.reflect.Field;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
@interface JsonProperty {
String value() default "";
}
class MyClass {
@JsonProperty("newName")
private String oldName;
private int age;
public MyClass(String oldName, int age) {
this.oldName = oldName;
this.age = age;
}
public String getOldName() {
return oldName;
}
public void setOldName(String oldName) {
this.oldName = oldName;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
public class Main {
public static void main(String[] args) {
MyClass obj = new MyClass("John", 25);
Field[] fields = obj.getClass().getDeclaredFields();
for (Field field : fields) {
String propertyName = field.getName(); // 默认属性名为字段名
if (field.isAnnotationPresent(JsonProperty.class)) { // 判断是否有 @JsonProperty 注解
JsonProperty annotation = field.getAnnotation(JsonProperty.class);
propertyName = annotation.value(); // 获取注解的值
}
field.setAccessible(true);
try {
Object propertyValue = field.get(obj); // 获取字段的值
System.out.println("Property Name: " + propertyName + ", Value: " + propertyValue);
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
}
```
在上面的示例中,`MyClass` 类中的 `oldName` 字段使用了 `@JsonProperty` 注解,将属性名改为了 "newName"。而 `age` 字段没有使用注解,仍然使用原属性名。
输出结果:
```
Property Name: newName, Value: John
Property Name: age, Value: 25
```
这样,根据注解的设置,可以灵活地改变类中属性名的输出。如果没有使用注解,默认输出原属性名。
阅读全文
相关推荐
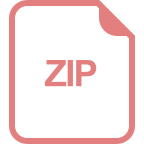

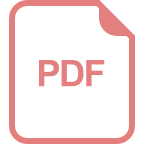


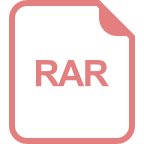
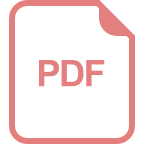
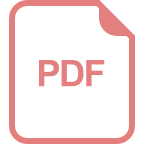
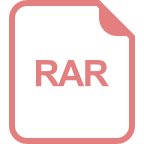
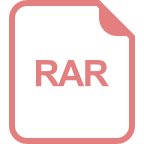
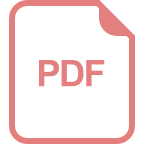
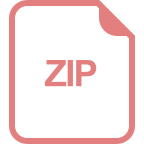
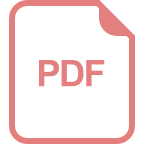
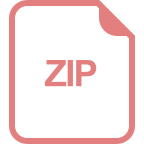
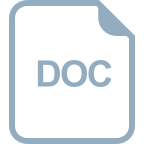
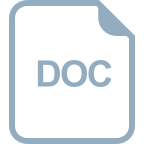