liunx 下线程优先级反转 的使用示例
时间: 2024-10-20 10:05:49 浏览: 1
在Linux系统中,线程优先级反转(Thread Priority Inversion)是指一个高优先级线程被阻塞,导致其后续运行的低优先级线程有机会临时提升其优先级并获得CPU资源的情况。这种现象通常是因为某些锁竞争或同步机制导致的。
一个简单的示例如下:
```bash
#include <pthread.h>
#include <semaphore.h>
#define MAX_THREADS 5
#define HIGH_PRIORITY 10
#define LOW_PRIORITY 5
typedef struct {
pthread_t thread;
int priority;
} ThreadData;
void *high_priority_func(void *arg) {
ThreadData *data = (ThreadData *) arg;
sem_wait(&lock); // 模拟获取某个需要同步的资源
data->priority = HIGH_PRIORITY;
pthread_setschedparam(pthread_self(), SCHED_FIFO, &data->priority);
printf("High priority thread started.\n");
sem_post(&lock); // 释放资源
return NULL;
}
void *low_priority_func(void *arg) {
ThreadData *data = (ThreadData *) arg;
while (1) {
sem_wait(&lock); // 阻塞等待
if (data->priority == LOW_PRIORITY) { // 如果变为低优先级,说明发生了优先级反转
printf("Priority inversion occurred, high priority thread is blocked.\n");
break;
}
sem_post(&lock); // 立即释放锁并继续循环
}
printf("Low priority thread exiting.\n");
return NULL;
}
int main() {
sem_t lock;
init_sem(&lock, 1); // 初始化互斥量
ThreadData threads[MAX_THREADS];
for (int i = 0; i < MAX_THREADS; i++) {
threads[i].priority = LOW_PRIORITY;
pthread_create(&threads[i].thread, NULL, low_priority_func, &threads[i]);
}
// 创建一个高优先级线程
threads[0].priority = HIGH_PRIORITY;
pthread_create(&threads[0].thread, NULL, high_priority_func, &threads[0]);
// 主函数不再阻塞
pthread_join(threads[0].thread, NULL);
return 0;
}
```
在这个示例中,`low_priority_func`会一直尝试获取锁,而`high_priority_func`在获取到锁后会将自身设置为高优先级。当`high_priority_func`因等待锁而被阻塞时,`low_priority_func`就会占据CPU时间,看似低优先级,但实际上由于高优先级线程被阻塞,就形成了优先级反转。
阅读全文
相关推荐
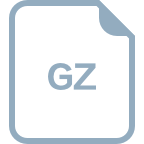
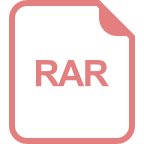
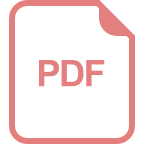


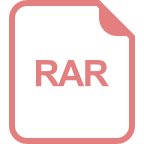
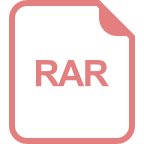
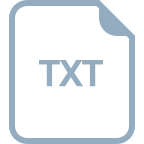
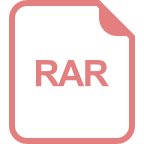
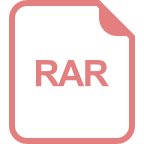
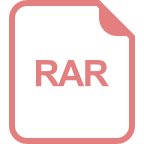
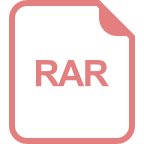
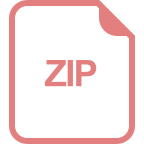
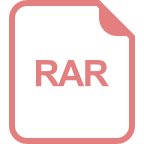
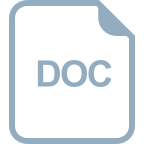
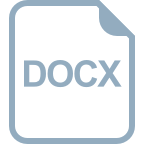
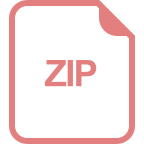