时间序列模型LSTM pytorch实现
时间: 2023-11-11 07:52:12 浏览: 126
LSTM(长短期记忆网络)是一种适用于处理序列数据的深度学习模型,它在处理时间序列数据时表现出色。在PyTorch中,我们可以使用torch.nn模块来实现LSTM模型。
以下是在PyTorch中实现LSTM时间序列模型的基本步骤:
1. 数据处理:首先,您需要准备和处理您的时间序列数据。这可能涉及到将原始数据转换为适合LSTM模型的张量形式,特征归一化以及数据集的划分等。
2. LSTM模型定义:接下来,您需要定义LSTM模型的结构。在PyTorch中,您可以使用torch.nn.LSTM类来定义LSTM模型。您需要指定输入维度,隐藏层维度,层数等参数。
3. 模型训练:使用定义好的LSTM模型,您可以将数据传递给模型进行训练。通过定义损失函数和优化器,您可以迭代地将数据送入模型中,计算损失,并使用优化器更新模型的参数。
4. 模型测试:训练完成后,您可以使用训练好的模型对新的数据进行预测和测试。通过将新的数据输入到模型中,您可以获得模型的输出,并进行后续的分析和评估。
关于LSTM模型在PyTorch中的更详细实现和代码示例,您可以参考引用和引用提供的资料。
相关问题
lstm pytorch实现
好的,关于使用 PyTorch 实现 LSTM,你可以按照以下步骤进行:
1. 导入 PyTorch 库和其他必要的库:
```python
import torch
import torch.nn as nn
```
2. 定义 LSTM 模型:
```python
class LSTM(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(LSTM, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size).to(device)
out, _ = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
```
其中,`input_size` 表示输入数据的特征数,`hidden_size` 表示 LSTM 隐藏层的大小,`num_layers` 表示 LSTM 层数,`output_size` 表示输出数据的大小。
3. 定义损失函数和优化器:
```python
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.Adam(model.parameters(), lr=learning_rate)
```
其中,`CrossEntropyLoss()` 是交叉熵损失函数,`Adam()` 是一种常用的优化器。
4. 训练模型:
```python
for epoch in range(num_epochs):
for i, (images, labels) in enumerate(train_loader):
images = images.reshape(-1, sequence_length, input_size).to(device)
labels = labels.to(device)
outputs = model(images)
loss = criterion(outputs, labels)
optimizer.zero_grad()
loss.backward()
optimizer.step()
if (i+1) % 100 == 0:
print('Epoch [{}/{}], Step [{}/{}], Loss: {:.4f}'
.format(epoch+1, num_epochs, i+1, total_step, loss.item()))
```
其中,`train_loader` 是训练数据的 DataLoader,`sequence_length` 表示序列长度,`total_step` 表示总共的步数。
5. 测试模型:
```python
with torch.no_grad():
correct = 0
total = 0
for images, labels in test_loader:
images = images.reshape(-1, sequence_length, input_size).to(device)
labels = labels.to(device)
outputs = model(images)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum().item()
print('Accuracy of the model on the test images: {} %'.format(100 * correct / total))
```
其中,`test_loader` 是测试数据的 DataLoader。
lstm pytorch
LSTM是一种循环神经网络,可以用于处理序列数据。在PyTorch中,可以使用nn.LSTM模块来构建LSTM网络。首先,需要导入必要的库和数据,例如torch和torch.nn。然后,可以加载数据并进行预处理,包括训练数据和测试数据的准备。接下来,可以搭建LSTM网络,定义网络的输入大小、隐藏层大小和隐藏层数量。最后,可以定义回归层网络,其输入维度等于LSTM的输出维度,输出维度为1。整个网络的结构可以通过forward方法来实现,其中需要将LSTM的输出进行reshape,然后通过回归层网络得到最终的输出。以下是用PyTorch实现LSTM网络的代码示例:
```python
import torch
from torch import nn
class RegLSTM(nn.Module):
def __init__(self, input_size, hidden_size, hidden_num_layers):
super(RegLSTM, self).__init__()
# 定义LSTM
self.rnn = nn.LSTM(input_size, hidden_size, hidden_num_layers)
# 定义回归层网络,输入的特征维度等于LSTM的输出,输出维度为1
self.reg = nn.Sequential(
nn.Linear(hidden_size, 1)
)
def forward(self, x):
x, (ht, ct) = self.rnn(x)
seq_len, batch_size, hidden_size = x.shape
x = x.view(-1, hidden_size)
x = self.reg(x)
x = x.view(seq_len, batch_size, -1)
return x
```
这段代码中,RegLSTM类继承了nn.Module类,重写了init和forward方法来构建LSTM网络。在init方法中,定义了LSTM和回归层网络的结构。在forward方法中,进行了LSTM网络的前向传播,并将输出进行了reshape。最后,返回了输出结果。这样就完成了使用PyTorch搭建LSTM网络的过程。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [人工智能(Pytorch)搭建模型2-LSTM网络实现简单案例](https://blog.csdn.net/weixin_42878111/article/details/129553278)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
- *3* [Pytorch实现的LSTM模型结构](https://blog.csdn.net/weixin_41744192/article/details/115270178)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
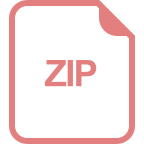








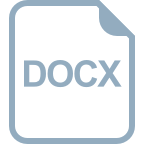
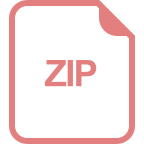



