用C语言写一个CRC的配置.c文件
时间: 2024-10-16 13:07:57 浏览: 18
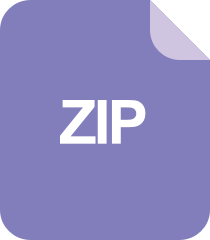
自己用C语言写的读取文件二进制数据,并计算CRC的程序
在C语言中,计算CRC(循环冗余校验)通常涉及到使用一些库函数或者自定义函数来生成和验证校验码。下面是一个简单的例子,创建了一个名为`crc_config.h`和`crc_config.c`的配置文件,包含了CRC-8的基本功能:
**crc_config.h**
```c
#ifndef CRC_CONFIG_H
#define CRC_CONFIG_H
#include <stdint.h>
// CRC-8 polynomial (0x07)
uint8_t crc8_poly = 0x07;
typedef uint8_t crc8_t;
// Function prototypes
crc8_t crc8_init(void);
crc8_t crc8_update(crc8_t crc, const uint8_t *data, size_t len);
crc8_t crc8_finalize(crc8_t crc);
#endif // CRC_CONFIG_H
```
**crc_config.c**
```c
#include "crc_config.h"
crc8_t crc8_init(void) {
return 0; // Initial value for the CRC register is typically zero
}
crc8_t crc8_update(crc8_t crc, const uint8_t *data, size_t len) {
for (size_t i = 0; i < len; ++i) {
crc ^= data[i];
for (int j = 0; j < 8; ++j) {
if (crc & 0x80) {
crc = (crc << 1) ^ crc8_poly;
} else {
crc <<= 1;
}
}
}
return crc;
}
crc8_t crc8_finalize(crc8_t crc) {
return crc ^ crc8_poly; // Apply final XOR with polynomial to get the checksum
}
```
这个配置文件包含了一个CRC-8算法的声明,初始化、数据更新以及最终化函数的实现。注意这只是一个基础示例,实际应用中可能需要根据所使用的CRC算法和位宽进行调整。
阅读全文
相关推荐
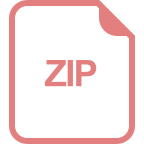
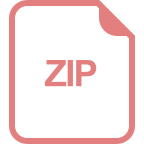
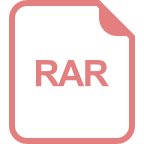
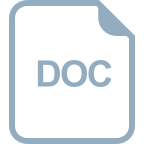
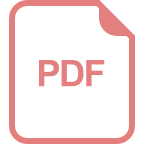
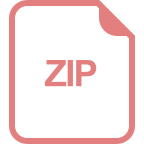
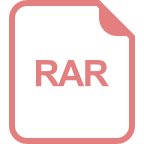
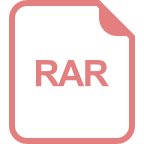
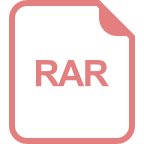
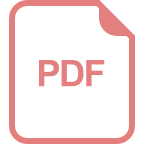
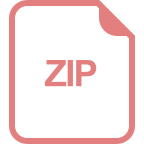
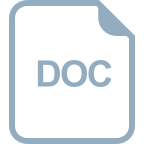
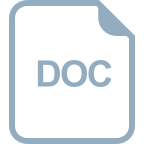
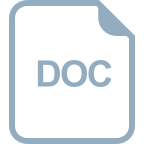
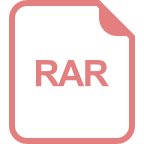
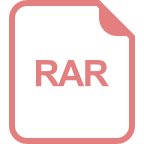
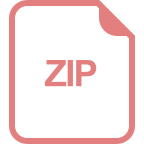
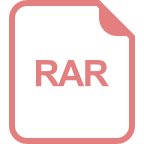