请将上述代码分成.h文件和.cpp文件写
时间: 2024-04-14 18:26:58 浏览: 108
以下是将示例代码分割成.h文件和.cpp文件的示例:
**widget.h**:
```cpp
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include <QGridLayout>
#include "draggablebutton.h"
class Widget : public QWidget
{
Q_OBJECT
public:
explicit Widget(QWidget *parent = nullptr);
private:
QGridLayout *gridLayout;
};
#endif // WIDGET_H
```
**widget.cpp**:
```cpp
#include "widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
{
gridLayout = new QGridLayout(this);
for (int row = 0; row < 2; ++row) {
for (int col = 0; col < 4; ++col) {
DraggableButton *button = new DraggableButton(this);
gridLayout->addWidget(button, row, col);
}
}
setLayout(gridLayout);
}
```
**draggablebutton.h**:
```cpp
#ifndef DRAGGABLEBUTTON_H
#define DRAGGABLEBUTTON_H
#include <QPushButton>
#include <QVBoxLayout>
#include <QGraphicsDropShadowEffect>
#include <QDrag>
#include <QMimeData>
#include <QMouseEvent>
class DraggableButton : public QPushButton
{
public:
explicit DraggableButton(QWidget *parent = nullptr);
protected:
void mousePressEvent(QMouseEvent *event) override;
void mouseMoveEvent(QMouseEvent *event) override;
private:
QPoint startPos;
};
#endif // DRAGGABLEBUTTON_H
```
**draggablebutton.cpp**:
```cpp
#include "draggablebutton.h"
DraggableButton::DraggableButton(QWidget *parent)
: QPushButton(parent)
{
setAcceptDrops(true);
setStyleSheet("QPushButton {border-radius: 10px;}");
QGraphicsDropShadowEffect *effect = new QGraphicsDropShadowEffect;
effect->setBlurRadius(10);
effect->setOffset(0);
effect->setColor(Qt::gray);
setGraphicsEffect(effect);
QLabel *label = new QLabel(this);
label->setAlignment(Qt::AlignCenter);
label->setText("Text");
QVBoxLayout *layout = new QVBoxLayout(this);
layout->addWidget(label);
setLayout(layout);
}
void DraggableButton::mousePressEvent(QMouseEvent *event)
{
if (event->button() == Qt::LeftButton) {
startPos = event->pos();
}
QPushButton::mousePressEvent(event);
}
void DraggableButton::mouseMoveEvent(QMouseEvent *event)
{
if (event->buttons() & Qt::LeftButton) {
int distance = (event->pos() - startPos).manhattanLength();
if (distance >= QApplication::startDragDistance()) {
QDrag *drag = new QDrag(this);
QMimeData *mimeData = new QMimeData;
drag->setMimeData(mimeData);
drag->exec();
}
}
QPushButton::mouseMoveEvent(event);
}
```
将代码分割成多个文件后,你可以在主函数(main.cpp)中使用以下代码进行调用:
```cpp
#include <QApplication>
#include "widget.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Widget widget;
widget.show();
return app.exec();
}
```
这样,代码就被分割成了多个文件,便于管理和维护。
希望这个示例对你有所帮助!如果还有其他问题,请随时提问。
阅读全文
相关推荐
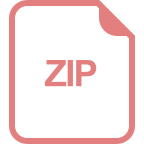
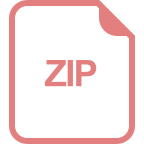



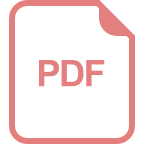
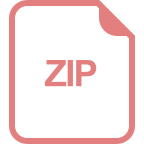
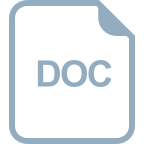
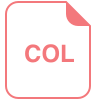
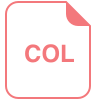
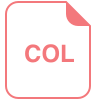
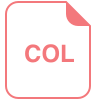
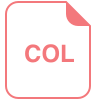
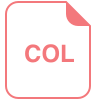
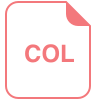
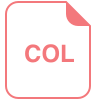

