springboot 从数据库把freemark加载到redis,并从redis获取模板完成赋值
时间: 2024-11-23 07:31:13 浏览: 9
在Spring Boot应用中,你可以利用Spring Data Redis和Thymeleaf模板引擎来实现这样的功能。首先,你需要配置Spring Data Redis以便连接和操作Redis数据库。这里是一个简化的步骤:
1. 添加依赖:在`pom.xml`或`build.gradle`文件中添加对Spring Data Redis和Freemarker的支持。
```xml
<!-- Maven -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring5</artifactId>
</dependency>
<!-- Gradle (Kotlin) -->
implementation('org.springframework.boot:spring-boot-starter-data-redis')
implementation('org.thymeleaf:thymeleaf-spring5')
```
2. 配置Redis:在`application.properties`或`application.yml`中设置Redis连接信息。
```properties
spring.redis.host=your_redis_host
spring.redis.port=6379
```
3. 创建数据源:如果你需要存储Freemarker模板,可以创建一个RedisTemplate并将其注入到服务中。
```java
@Configuration
public class RedisConfig {
@Bean
public StringRedisTemplate stringRedisTemplate(RedisConnectionFactory factory) {
return new StringRedisTemplate(factory);
}
}
```
4. 将Freemarker模板存储到Redis:在你的业务逻辑中,读取数据库的数据然后使用`StringRedisTemplate.opsForValue()`方法将模板内容保存到Redis。
```java
@Autowired
private StringRedisTemplate redisTemplate;
// 示例方法,假设你有DatabaseService用于查询数据库
public void storeTemplate(String templateKey, String templateContent) {
redisTemplate.opsForValue().set(templateKey, templateContent);
}
```
5. 获取并渲染模板:当需要使用模板时,从Redis中获取内容,通过`Thymeleaf`引擎完成变量的替换和渲染。
```java
@GetMapping("/render")
public String renderFromRedis(@RequestParam String key) {
String templateContent = redisTemplate.opsForValue().get(key); // 从Redis获取模板
Map<String, Object> model = new HashMap<>();
// ...填充模型数据
return new ThymeleafViewResolver().resolveTemplateIntoModel(model, "yourTemplateName", request).getContentAsString();
}
```
阅读全文
相关推荐
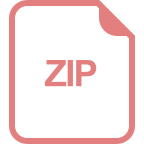
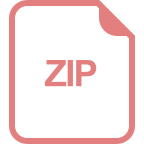
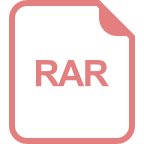

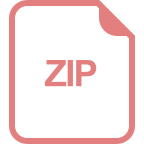
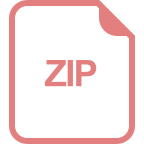
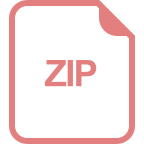
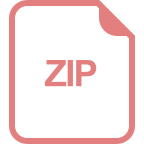
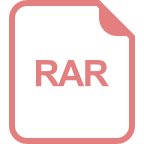
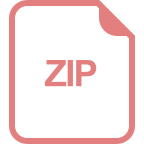
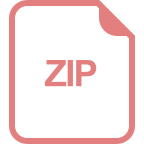
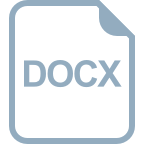
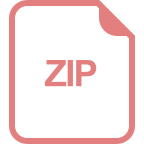
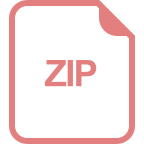
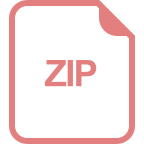
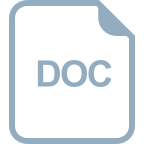


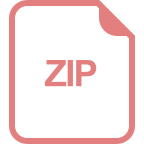