如何修改Person类,将其成员变量更改为表示出生日期?并且,请说明应如何设计一个age()方法来计算并返回个人的当前年龄?
时间: 2024-12-19 09:19:17 浏览: 3
为了将`Person`类的成员变量改为表示出生日期,你可以考虑将它从`String`或其他类型改为`Date`或者`LocalDate`,这取决于具体的日期需求和库支持。这里假设我们使用`java.util.LocalDate`:
```java
import java.time.LocalDate;
public class Person {
private LocalDate birthDate; // 更改为出生日期
// 构造函数
public Person(LocalDate birthDate) {
this.birthDate = birthDate;
}
// 计算当前年龄的age()方法(这里使用Java 8+的API)
public int getAge() {
LocalDate currentDate = LocalDate.now(); // 获取当前日期
Period agePeriod = Period.between(birthDate, currentDate); // 计算年龄差值
return agePeriod.getYears(); // 返回年龄(年数)
}
}
```
在这个例子中,`getAge()`方法会计算出生日期到现在的年份差,得到的就是个人的实际年龄。需要注意的是,如果生日还没过,实际年龄会比当前显示的少一岁。
相关问题
定义一个名为employee的类,它继承person类,其中定义salary(表示工资)和department(表示部门)两个成员变量和封装这两个变量的方法。编写主程序检查新建类中的所有方法。
好的,下面是用中文回答您的问题:
首先,我们可以定义一个名为Employee的类,它继承自Person类。在Employee类中,我们可以定义两个变量:salary和department,分别表示工资和部门。我们可以使用以下代码来定义这个类:
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
class Employee(Person):
def __init__(self, name, age, salary, department):
super().__init__(name, age)
self.salary = salary
self.department = department
def change_salary(self, new_salary):
self.salary = new_salary
def change_department(self, new_department):
self.department = new_department
```
在这个类中,我们定义了一个构造函数,它接收name、age、salary和department四个参数。在构造函数中,我们首先调用父类的构造函数来初始化name和age属性。然后,我们初始化salary和department属性。最后,我们定义了两个方法,change_salary和change_department,用于更改工资和部门属性。
现在,我们已经定义了Employee类,接下来我们可以创建一个新的对象,并使用它的方法来更改属性。例如:
```python
employee = Employee("张三", 25, 5000, "销售部")
print(employee.salary) # 输出:5000
employee.change_salary(6000)
print(employee.salary) # 输出:6000
print(employee.department) # 输出:销售部
employee.change_department("市场部")
print(employee.department) # 输出:市场部
```
在上面的代码中,我们首先创建了一个名为employee的Employee对象,并输出了它的初始工资值。然后,我们使用change_salary方法将工资值更改为6000,并输出了新的工资值。接下来,我们输出了部门属性的初始值,并使用change_department方法将其更改为“市场部”,最后再次输出了部门属性的值。
声明一个Person类,在其中定义一个私有变量age,通过定义Age属性设定age变量的输出范围为0~150,若超出这个范围,则age值为18.
在Java中,你可以通过创建一个名为`Person`的类,并使用访问修饰符(如`private`)来声明一个私有变量`age`。为了限制年龄在0到150的范围内,你可以在类中定义一个getter方法(也称为accessor method)以及一个setter方法(mutator method),并在setter方法中添加验证逻辑。
下面是一个示例:
```java
public class Person {
// 私有变量,年龄范围限定0-150
private int age;
// 定义getAge()方法获取年龄,返回默认值18如果超过范围
public int getAge() {
if (age < 0 || age > 150) {
return 18;
}
return age;
}
// 定义setAge(int newAge)方法设置年龄,添加范围检查
public void setAge(int newAge) {
if (newAge >= 0 && newAge <= 150) {
this.age = newAge;
} else {
System.out.println("警告:年龄超出了0-150的范围,已设为18岁");
this.age = 18;
}
}
}
```
在这个例子中,如果你试图设置一个超出范围的年龄,程序会打印一条警告消息并将年龄更改为18。当你通过`Person`对象获取`age`时,实际显示的将是18或用户输入的有效范围内的值。
阅读全文
相关推荐
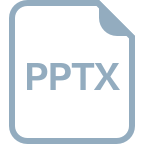
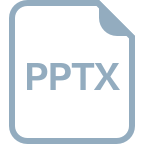
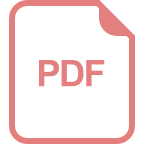
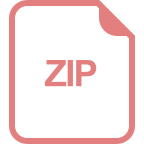
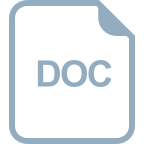
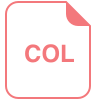
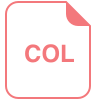
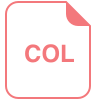
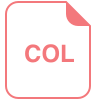
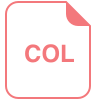
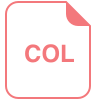





