int CR_RegisterEventCallback (int nDetrIdx, ICallback∗ pCallback ) ; class ICallback { public: ICallback () {}; virtual ˜ ICallback () {}; void virtual Process (int nEventID, CR Event∗ pEvent) {}; }; enum CR_EventID { CR EVT SERVER DISCONNECTED, // dropped connection with server CR EVT DETR DISCONNECTED, // dropped connection with detector CR EVT TEMPERATURE INFO, // temperature of the detector CR EVT NEW FRAME, // Arrival of a new frame CR EVT CALIBRATION IN PROGRESS, // Calibration in progress CR EVT CALIBRATION FINISHED, // Completion of calibration CR EVT ACQ STAT INFO // Acquisition of statistical summary }; struct CR_Event { int nDetrIdx; int nWidth; // Same as CR ModeInfo.nImageWidth, see A.4 int nHeight; int nPixelDepth; void∗ pData; }; struct CR_AcquisitionStatInfo { int nTotalFrameNum; // Total number of frames acquired int nLostFrameNum; // Number of lost frames float fStatFrameRate; float fTransmissionSpeed; long long nAcqDuration; // Duration of image acquisition } python调用回调函数CCallbackImp∗ pCallback = new CCallbackImp(); CR RegisterEventCallback(cDETR IDX, pCallback);
时间: 2024-04-20 19:27:46 浏览: 182
在Python中调用C++的回调函数时,你可以使用ctypes库。首先,你需要将C++的函数指针转换为Python的回调函数类型。然后,你可以通过调用C++函数`CR_RegisterEventCallback`来注册回调函数。
下面是一个简单的示例代码:
```python
import ctypes
# 定义回调函数类型
CallbackType = ctypes.CFUNCTYPE(None, ctypes.c_int, ctypes.POINTER(CR_Event))
# 定义回调函数
def callback(nEventID, pEvent):
# 在这里实现回调函数的逻辑
print("Callback function called")
# ...
# 创建回调函数对象
callback_obj = CallbackType(callback)
# 加载C++库
cpp_lib = ctypes.CDLL("your_library.dll")
# 创建CCallbackImp对象
pCallback = cpp_lib.CCallbackImp()
# 注册回调函数
CR_RegisterEventCallback = cpp_lib.CR_RegisterEventCallback
CR_RegisterEventCallback.argtypes = [ctypes.c_int, ctypes.POINTER(ICallback)]
CR_RegisterEventCallback.restype = ctypes.c_int
# 调用注册函数
nDetrIdx = cDETR_IDX # 设置参数值
result = CR_RegisterEventCallback(nDetrIdx, ctypes.pointer(pCallback))
# 检查注册结果
if result != 0:
print("Failed to register callback")
```
在这个示例中,`your_library.dll`是你的C++代码编译生成的动态链接库文件,你需要将其替换为正确的文件名。然后,你可以根据实际需要修改回调函数的逻辑。
希望这个示例能够帮助你实现在Python中调用C++的回调函数。如果有任何问题,请随时提问。
阅读全文
相关推荐
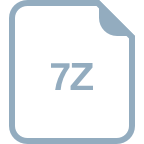
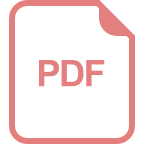
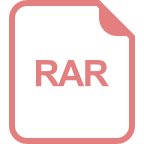


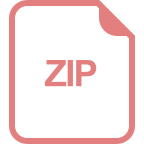
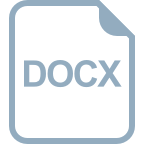
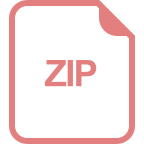
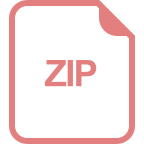
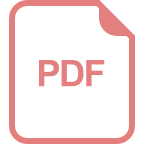