flutter 子组件如何调用父组件方法
时间: 2024-08-12 10:10:26 浏览: 147
在 Flutter 中,子组件(通常是一个 Widget)要调用父组件的方法,可以通过以下几种方式:
1. **通过`InheritedWidget`**:如果你的父组件实现了某个 `InheritedWidget`,子组件可以通过 `InheritedData` 获取到父组件的数据,并通过该数据调用父组件的函数。例如:
```dart
class Parent extends StatelessWidget with InheritedWidget<ParentData> {
@override
ParentData get data => _data;
// 父组件方法
void parentFunction() {
// ...
}
@override
Widget build(BuildContext context) {
return Child(
// 在Child中调用父方法
parentFunction(),
);
}
}
// 子组件 Child
class Child extends StatelessWidget {
@override
Widget build(BuildContext context) {
// 如果需要调用父组件方法,使用 context.inheritedWidget.parentFunction();
}
}
```
2. **通过事件总线(Event Bus)**:如果子组件和父组件间没有直接的继承关系,可以使用事件总线如 `Provider` 或 `Riverpod` 来传递消息,父组件发布事件,子组件订阅并处理。
3. **回调函数(Callback)**:在构建子组件时,将需要调用的父组件方法作为参数传递。例如,使用 `onTap`、`onChanged` 等回调:
```dart
class Parent extends StatefulWidget {
final Function(String) onValueChange;
@override
_ParentState createState() => _ParentState();
}
class _ParentState extends State<Parent> {
void handleValueChange(String value) {
// 父组件处理方法
}
@override
Widget build(BuildContext context) {
return Container(
child: RaisedButton(
onPressed: () {
// 触发回调
handleValueChange("from Child");
},
child: Text('Click me'),
),
);
}
}
// 子组件
class Child extends StatelessWidget {
@override
Widget build(BuildContext context) {
return RaisedButton(
onPressed: () {
// 调用父组件方法,传递参数
widget.onValueChange("from Child");
},
child: Text('Click me too'),
);
}
}
```
相关问题--:
1. 如何在 Flutter 中使用 `InheritedWidget` 实现父子组件通信?
2. 什么是事件总线?在 Flutter 中如何使用它们来传递方法调用?
3. 何时会使用回调函数作为参数传递给子组件?
阅读全文
相关推荐
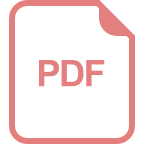
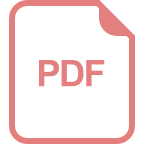









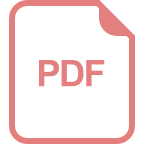
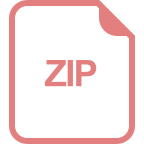
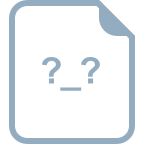
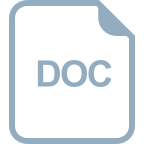


