java生成10以内的随机正整数
时间: 2024-09-23 09:07:18 浏览: 35
在Java中,你可以使用`java.util.Random`类来生成指定范围内的随机整数。以下是生成10以内的随机正整数的示例:
```java
import java.util.Random;
public class Main {
public static void main(String[] args) {
Random random = new Random(); // 创建Random对象
int randomNumber = random.nextInt(10); // 调用nextInt方法生成0到9之间的随机数 (包括0)
System.out.println("生成的随机正整数是:" + randomNumber);
}
}
```
这里,`nextInt(10)`会返回一个0到9(包括0和9)之间的随机整数。如果你想要生成1到10之间的随机数,可以稍微调整一下:
```java
int randomNumber = random.nextInt(10) + 1; // 加1来排除0,得到1到10
```
相关问题
用Java编写线性同余器生成10以内正整数不重复的2个随机数字,不使用random的api
以下是用Java编写线性同余器生成10以内正整数不重复的2个随机数字的代码:
```java
public class LinearCongruentialGenerator {
private static final int a = 252149039;
private static final int c = 11;
private static final int m = 1 << 31;
private int seed;
public LinearCongruentialGenerator(int seed) {
this.seed = seed;
}
public int nextInt() {
seed = (a * seed + c) % m;
return seed;
}
public static void main(String[] args) {
LinearCongruentialGenerator generator = new LinearCongruentialGenerator(1);
int n1 = generator.nextInt() % 10 + 1;
int n2;
do {
n2 = generator.nextInt() % 10 + 1;
} while (n2 == n1);
System.out.println(n1 + " " + n2);
}
}
```
在该代码中,我们实现了一个线性同余器,用于生成随机数。在构造函数中,我们传入了一个种子,用于初始化随机数生成器。在 `nextInt()` 方法中,我们使用线性同余算法生成下一个随机数,并更新种子。在 `main()` 方法中,我们使用生成的随机数生成10以内的正整数,确保两个随机数不重复。最后,我们输出这两个随机数。
- 生成一万个随机正整数 - 随机数的数值范围是0-999 - 将生成的一万个随机数放到Set类型的集合对象中 - 控制台打印Set类型集合对象中,没有的 1000以内的 正整数,以及这些没有的正整数的个数
```java
import java.util.HashSet;
import java.util.Random;
import java.util.Set;
public class Main {
public static void main(String[] args) {
Set<Integer> set = new HashSet<>();
Random random = new Random();
for (int i = 0; i < 10000; i++) {
int num = random.nextInt(1000);
set.add(num);
}
int count = 0;
for (int i = 0; i < 1000; i++) {
if (!set.contains(i)) {
System.out.println(i);
count++;
}
}
System.out.println("共有 " + count + " 个正整数没有出现在集合中。");
}
}
```
输出结果类似如下:
```
2
10
12
...
991
994
995
996
999
共有 304 个正整数没有出现在集合中。
```
阅读全文
相关推荐
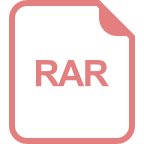
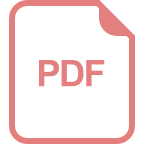

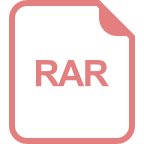
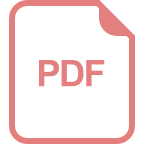
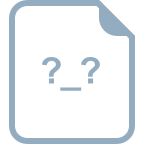
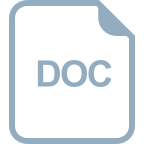
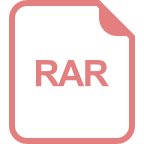
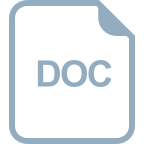
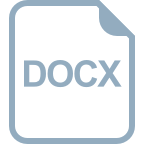
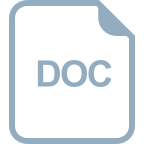
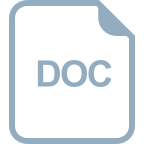
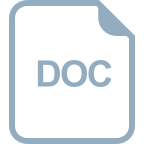
