c语言 输入某学生的数学( math)、英语( english)和 C 语言(C)的成绩, 输出该学生这三门课的成绩、总成绩( sum)和平均成绩( aver)
时间: 2024-11-25 14:15:24 浏览: 26
在C语言中,你可以通过使用结构体来存储学生的成绩信息,并编写函数来获取用户输入并计算总分和平均分。首先,定义一个包含三个成员变量的结构体:
```c
typedef struct {
int math;
int english;
int C;
} StudentScore;
```
然后编写主程序,包括获取输入、创建结构体实例以及计算总分和平均分:
```c
#include <stdio.h>
void input_score(StudentScore* score) {
printf("请输入学生的数学成绩:");
scanf("%d", &score->math);
printf("请输入学生的英语成绩:");
scanf("%d", &score->english);
printf("请输入学生的C语言成绩:");
scanf("%d", &score->C);
}
int calculate_sum_and_average(StudentScore score) {
int sum = score.math + score.english + score.C;
double average = (double)sum / 3; // 防止整数除法溢出
return average;
}
int main() {
StudentScore student;
// 获取输入
input_score(&student);
// 计算总分和平均分
int total = calculate_sum_and_average(student);
double avg = calculate_sum_and_average(student); // 双精度平均值
printf("该学生三门课程的成绩分别是:\n"
"数学: %d\n"
"英语: %d\n"
"C语言: %d\n",
student.math, student.english, student.C);
printf("总成绩: %d\n"
"平均成绩: %.2f\n", total, avg); // 控制小数点后两位
return 0;
}
```
在这个程序中,`input_score` 函数用于从用户那里获取分数,`calculate_sum_and_average` 函数则计算总分和平均分。记得运行这个程序时,在控制台依次输入学生的三门课程成绩。
阅读全文
相关推荐
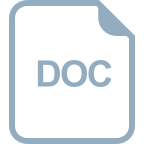
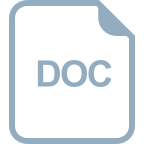
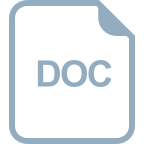














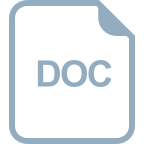
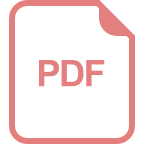
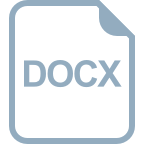