解压rar和zip java
时间: 2024-10-16 17:03:18 浏览: 28
在Java中,要解压RAR和ZIP文件,可以利用一些第三方库,比如Apache Commons Compress库。这个库提供了一组工具类来处理各种常见的压缩格式,包括RAR和ZIP。
以下是使用`commons-compress`库来解压RAR和ZIP文件的一个简单示例:
```java
import org.apache.commons.compress.archivers.ArchiveEntry;
import org.apache.commons.compress.archivers.ArchiveException;
import org.apache.commons.compress.archivers.RarArchiveEntry;
import org.apache.commons.compress.archivers.zip.ZipArchiveEntry;
import org.apache.commons.compress.utils.IOUtils;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardCopyOption;
public class FileUnpacker {
public static void main(String[] args) {
String archivePath = "path_to_your_archive.rar/zip"; // 替换为你的文件路径
String outputPath = "output_directory"; // 输出目录
try (FileArchiveInputStream in = new FileArchiveInputStream(new File(archivePath))) {
ArchiveStreamHandler handler = null;
if (in instanceof RarArchiveInputStream) {
handler = new RarArchiveStreamHandler();
} else if (in instanceof ZipArchiveInputStream) {
handler = new ZipArchiveStreamHandler();
}
if (handler != null) {
while ((entry = handler.nextEntry()) != null) {
String entryName = entry.getName();
Path outputFile = outputPath + "/" + entryName;
Files.createDirectories(outputFile.getParent());
IOUtils.copy(in, Files.newOutputStream(outputFile), StandardCopyOption.REPLACE_EXISTING);
}
}
} catch (IOException | ArchiveException e) {
System.err.println("Error occurred while extracting: " + e.getMessage());
}
}
}
```
在这个例子中,首先检查输入流是否为RAR或ZIP,然后创建对应的档案流处理器,逐个解压每个条目到指定的输出目录。记得先添加`<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-compress</artifactId>
<version>版本号</version>
</dependency>`到你的项目Maven依赖中,替换`版本号`为你需要的具体版本。
阅读全文
相关推荐
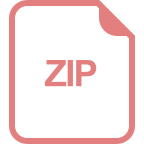
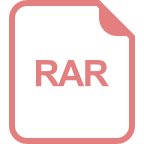
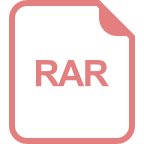
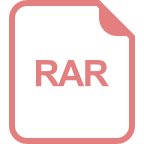
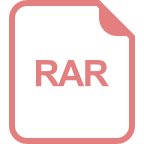
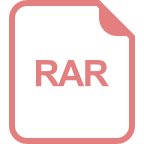
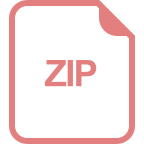
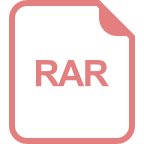
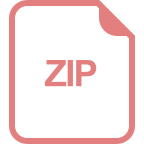
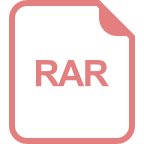
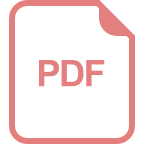
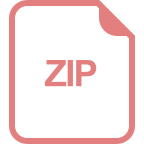
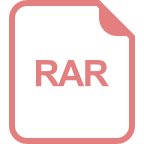
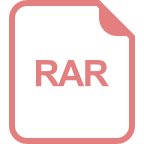
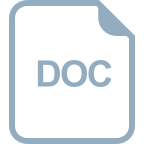
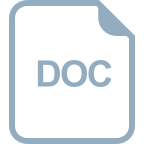
