c++ string用法
时间: 2024-03-03 22:45:56 浏览: 88
C++中的string是一个非常常用的字符串类,它提供了许多方便的方法来处理字符串。下面是一些C++ string的常见用法:
1. 创建string对象:
可以使用以下方式创建一个string对象:
```cpp
std::string str; // 创建一个空字符串
std::string str = "Hello"; // 创建一个包含初始值的字符串
std::string str("Hello"); // 使用构造函数创建字符串
```
2. 获取字符串长度:
可以使用`length()`或`size()`方法获取字符串的长度:
```cpp
std::string str = "Hello";
int len = str.length(); // 或者使用 str.size();
```
3. 字符串连接:
可以使用`+`运算符或`append()`方法将两个字符串连接起来:
```cpp
std::string str1 = "Hello";
std::string str2 = "World";
std::string result = str1 + " " + str2; // 使用 + 运算符
std::string result = str1.append(" ").append(str2); // 使用 append() 方法
```
4. 字符串比较:
可以使用`==`、`!=`、`<`、`>`、`<=`、`>=`等运算符来比较两个字符串的大小:
```cpp
std::string str1 = "Hello";
std::string str2 = "World";
if (str1 == str2) {
// 字符串相等
} else if (str1 < str2) {
// str1 小于 str2
} else {
// str1 大于 str2
}
```
5. 字符串查找和替换:
可以使用`find()`方法查找子字符串在字符串中的位置,使用`replace()`方法替换字符串中的子字符串:
```cpp
std::string str = "Hello World";
int pos = str.find("World"); // 查找子字符串的位置
str.replace(pos, 5, "C++"); // 替换子字符串
```
6. 字符串切割:
可以使用`substr()`方法从字符串中提取子字符串:
```cpp
std::string str = "Hello World";
std::string sub = str.substr(6, 5); // 提取子字符串,从位置6开始,长度为5
```
阅读全文
相关推荐
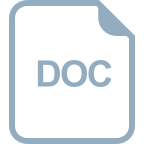
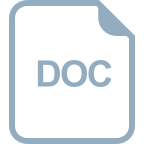
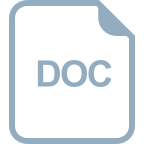

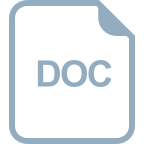
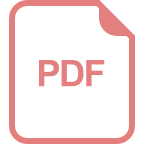
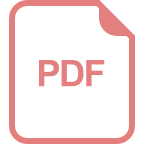
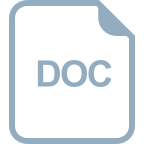
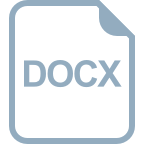
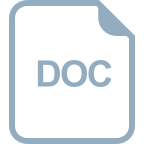
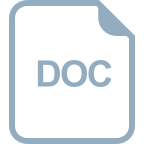



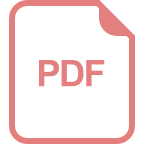



