已经通过staruml画出类图了,再通过主函数,从键盘对学生对象的数组(全班学生信息)进行输入,然后求出每个学生的总成绩和平均成绩,并显示全班学生的总成绩最高者的全部数据信息
时间: 2024-09-30 14:14:08 浏览: 32
首先,我们需要理解你的需求是在使用StarUML创建了一个类图的基础上,编写程序来处理用户输入的学生信息并计算相关统计数据。这个过程通常涉及以下几个步骤:
1. **读取学生对象**:从主函数开始,你需要创建一个动态数组或列表来存储学生对象,可以假设每个学生有姓名、各科成绩等属性。
```python
# 假设我们有一个Student类
class Student:
def __init__(self, name, scores):
self.name = name
self.scores = scores
def input_students():
students_list = []
# 用户输入,比如按分隔符逗号或换行
user_input = input("请输入全班学生信息,格式如:张三,数学:85,语文:90...")
for line in user_input.split("\n"):
student_data = line.split(",")
name = student_data[0]
scores_str = student_data[1:]
scores = [int(score) for score in scores_str] # 将字符串转换为整数
students_list.append(Student(name, scores))
return students_list
```
2. **计算总成绩和平均成绩**:遍历学生列表,对每个学生进行操作。
```python
def calculate_totals(student_list):
total_scores = [sum(student.scores) for student in student_list]
average_scores = [total / len(student.scores) for total in total_scores]
max_total_student = max(student_list, key=lambda x: sum(x.scores))
return total_scores, average_scores, max_total_student
```
3. **展示结果**:最后,打印出每个学生的总成绩、平均成绩以及总成绩最高的学生详细信息。
```python
def display_results(total_scores, average_scores, max_total_student):
print(f"总成绩:{total_scores}")
print(f"平均成绩:{average_scores}")
print(f"总成绩最高者:")
print(f"姓名:{max_total_student.name}")
print(f"各科成绩:{max_total_student.scores}")
# 调用以上函数
students_list = input_students()
total, avg, max_student = calculate_totals(students_list)
display_results(total, avg, max_student)
```
如果你已经创建了Student类并且类图设计合理,只需要将上述代码整合到你的项目中即可满足需求。如果还有疑问,关于如何在星形图中组织类或如何更好地结构化代码,可以根据类图调整。
阅读全文
相关推荐
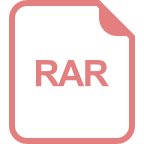
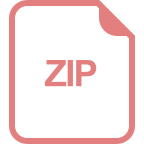
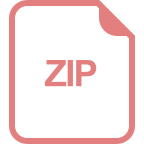

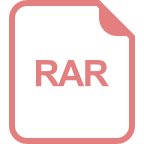
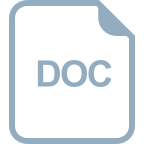
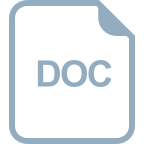


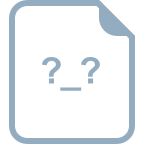
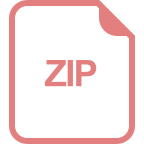
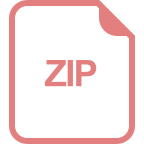
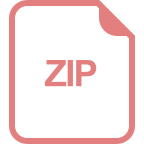