使用 mvc 编写水果类从商品类中继承
时间: 2024-10-24 16:03:12 浏览: 10
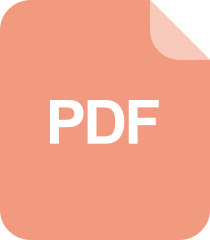
django商品分类及商品数据建模实例详解
在MVC(Model-Controller)设计模式中,如果你想要编写一个关于"水果"的类,并让它从更通用的"商品"类中继承,通常步骤如下:
1. **创建基础模型 (Model)**: 首先,定义一个名为`Product`或`Item`的基类,作为所有商品的抽象表示,包含共同属性如名称、价格等。
```java
public abstract class Product {
private String name;
private double price;
// 构造函数、getter和setter方法
public Product(String name, double price) {
this.name = name;
this.price = price;
}
// getters and setters
public String getName() { return name; }
public void setName(String name) { this.name = name; }
public double getPrice() { return price; }
public void setPrice(double price) { this.price = price; }
}
```
2. **创建特定模型 (Subclass)**: 然后创建一个名为`Fruit`的具体类,它继承自`Product`,并可能添加一些水果特有的属性或方法,比如种类、季节等。
```java
public class Fruit extends Product {
private String type;
private Season season;
public Fruit(String name, double price, String type, Season season) {
super(name, price);
this.type = type;
this.season = season;
}
// getters and setters for fruit-specific properties
public String getType() { return type; }
public void setType(String type) { this.type = type; }
public Season getSeason() { return season; }
public void setSeason(Season season) { this.season = season; }
}
```
3. **控制器 (Controller) 和视图 (View)**: 控制器负责处理用户的请求和操作,它可以实例化`Fruit`对象并传递给视图展示。视图则根据模型提供的信息显示内容。
在这个例子中,`Controller`可能会有一个方法接收用户输入的水果信息,然后创建一个`Fruit`对象,并通过`View`展示出来。
阅读全文
相关推荐
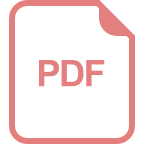
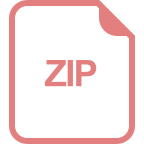
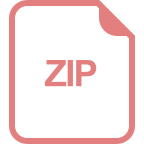
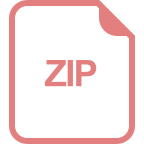
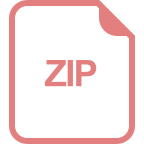
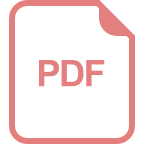
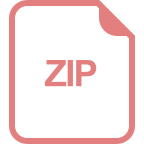
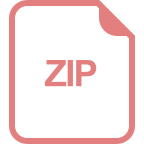
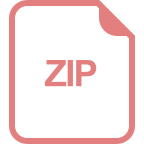
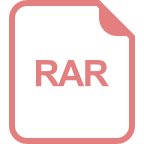
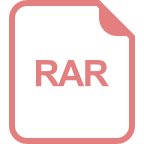
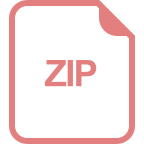
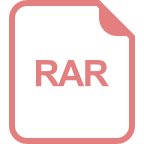
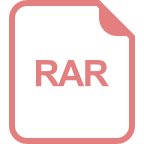
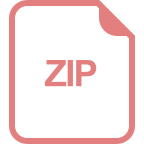
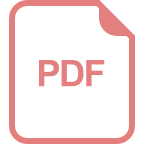
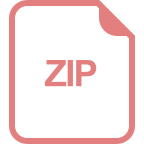