C# radioButton.Tag
时间: 2023-11-23 10:58:09 浏览: 163
在C#中,RadioButton控件有一个Tag属性,可以用来存储与该控件相关的任何对象。Tag属性通常用于存储控件的自定义数据,以便在运行时使用。例如,您可以将一个对象分配给RadioButton的Tag属性,然后在单击RadioButton时访问该对象。
以下是一个示例,演示如何使用RadioButton的Tag属性:
```csharp
// 创建一个名为radioButton1的RadioButton控件
RadioButton radioButton1 = new RadioButton();
// 将一个字符串分配给radioButton1的Tag属性
radioButton1.Tag = "这是一个字符串";
// 在单击radioButton1时,显示radioButton1的Tag属性值
private void radioButton1_CheckedChanged(object sender, EventArgs e)
{
MessageBox.Show(radioButton1.Tag.ToString());
}
```
在上面的示例中,当单击radioButton1时,将显示一个消息框,其中包含字符串“这是一个字符串”。
相关问题
c# WINFORM 选框选中后加入到缓存并在在其它程序类库实时可以调用
在 WinForms 程序中,如果你想在单选按钮(RadioButton)或复选框(CheckBox)选中后将其状态加入缓存,并允许其他程序类库(如独立的业务逻辑层或第三方API)实时访问,你可以按照以下步骤操作:
1. **创建缓存管理类**:
创建一个单独的类,例如 `CacheManager`,用于存储选中的控件状态。这里我们可以使用 `ConcurrentDictionary` 作为缓存容器,确保线程安全。
```csharp
using System.Collections.Concurrent;
public static class CacheManager
{
private static readonly ConcurrentDictionary<string, object> StateCache = new ConcurrentDictionary<string, object>();
public static void SaveState(string key, object state)
{
StateCache[key] = state;
}
public static object LoadState(string key)
{
return StateCache.TryGetValue(key, out var value) ? value : default;
}
}
```
2. **WinForms Form 上的事件处理**:
在 Form 的加载或复选框的 Click 事件中,保存选中的状态到缓存。
```csharp
private void radioButton_CheckedChanged(object sender, EventArgs e)
{
RadioButton radioButton = (RadioButton)sender;
if (radioButton.Checked)
{
CacheManager.SaveState(radioButton.Name, radioButton.Text);
}
}
private void checkBox_CheckedChanged(object sender, EventArgs e)
{
CheckBox checkBox = (CheckBox)sender;
if (checkBox.Checked)
{
CacheManager.SaveState(checkBox.Name, checkBox.Tag); // 如果有自定义标签
}
}
```
3. **其他程序类库访问**:
其他类库想要访问缓存,只需引入相同的 `CacheManager` 类,然后像下面这样获取状态。
```csharp
// 在另一个类中
public static string GetRadioButtonSelection()
{
return CacheManager.LoadState("RadioButtonKey") as string;
}
public static object GetCheckBoxSelection(string checkBoxId)
{
return CacheManager.LoadState(checkBoxId);
}
```
注意:以上代码假设你已经有了明确的键(如 `RadioButtonKey` 和 `checkBoxId`),如果需要动态生成键,则需要相应地调整。
4. **跨线程访问**:
如果在不同线程中访问缓存,确保使用 `ConcurrentDictionary` 的特性,它能够在线程安全的情况下操作。
现在,选中的控件状态就被保存到缓存中,其他程序类库可以实时地调用 `GetRadioButtonSelection` 和 `GetCheckBoxSelection` 来获取状态。记得在使用缓存之前检查键是否存在,因为默认情况下 `LoadState` 返回的是 `default` 值。
阅读全文
相关推荐








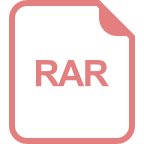



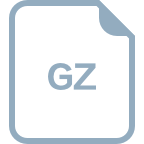
