C语言,输入一连串数字,个数末知,把数字串中的数字按从小到大排列后输出
时间: 2024-12-10 12:56:37 浏览: 11
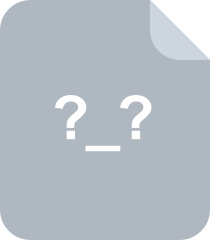
输入三个数字,按从大到小输出 C语言代码
在C语言中,你可以使用动态内存分配和循环结构来处理这种无固定长度的数字序列。一种常见的方法是利用`fgets()`函数读取一行字符串,然后逐字符解析出整数并存储在一个数组或链表中。接下来,你可以使用快速排序、冒泡排序或其他合适的排序算法对数组进行排序,最后再遍历排序后的数组并打印结果。
下面是一个简单的示例,使用数组来存储输入的数字,并使用选择排序进行排序:
```c
#include <stdio.h>
#include <stdlib.h>
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
void selection_sort(int arr[], int n) {
for (int i = 0; i < n - 1; ++i) {
int min_idx = i;
for (int j = i + 1; j < n; ++j) {
if (arr[j] < arr[min_idx]) {
min_idx = j;
}
}
swap(&arr[i], &arr[min_idx]);
}
}
int main() {
char input[100];
int num, count = 0;
// 读取数字直到用户输入'\n'
while (scanf("%d", &num) == 1 && num != 0) {
input[count++] = num;
input[count] = '\0'; // 添加换行符终止输入
}
input[count] = '\0';
// 将字符串转换为整数数组并排序
int *sorted_arr = malloc(sizeof(int) * count);
sscanf(input, "%d", sorted_arr);
selection_sort(sorted_arr, count);
// 打印排序后的数字
printf("Sorted numbers: ");
for (int i = 0; i < count; ++i) {
printf("%d ", sorted_arr[i]);
}
free(sorted_arr); // 别忘了释放动态分配的内存
return 0;
}
```
阅读全文
相关推荐
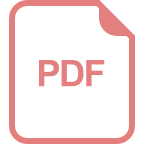
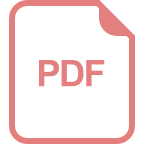
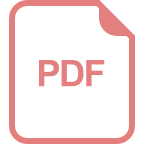
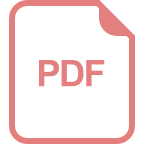
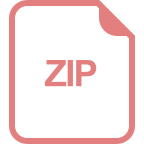
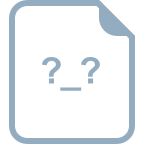
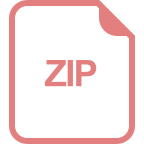
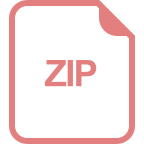
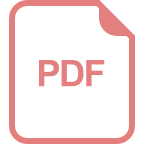
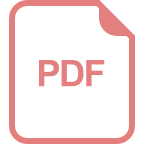
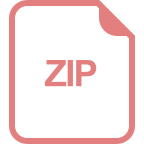
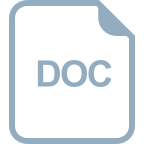
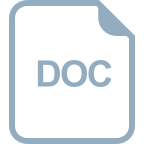