人物卡通化python代码
时间: 2024-09-02 09:00:23 浏览: 59
在Python中,我们可以使用PIL(Python Imaging Library)或者其他图像处理库如OpenCV、Pillow等来实现简单的字符卡通化效果。这里以Pillow为例,提供一个基本的卡通化过程,它通常涉及到颜色调整和滤镜应用。
```python
from PIL import Image, ImageFilter
def cartoonify(image_path):
# 打开图片
img = Image.open(image_path)
# 将图片转为灰度,减少颜色信息,便于卡通化处理
gray_img = img.convert('L')
# 应用边缘增强滤镜,突出线条
enhanced_img = gray_img.filter(ImageFilter.EDGE_ENHANCE_MORE)
# 使用模糊滤镜,添加卡通风格的像素感
cartoon_img = enhanced_img.filter(ImageFilter.BLUR)
# 返回卡通化的图片
return cartoon_img
# 示例用法
input_image = 'input.jpg'
output_image = 'cartoonized_' + input_image
cartoonify(input_image).save(output_image)
相关问题
画卡通人物帕恰狗的python代码
以下是画帕恰狗的Python代码:
```python
import turtle
# 设置画笔
t = turtle.Turtle()
.speed(10)
t.pensize(5)
# 画身体
t.fillcolor('#F5DEB3')
t.begin_fill()
t.circle(100)
t.end_fill()
# 画头部
t.penup()
t.goto(0, 150)
t.pendown()
t.fillcolor('#F5DEB3')
t.begin_fill()
t.circle(50)
t.end_fill()
# 画眼睛
t.penup()
t.goto(-30, 180)
t.pendown()
t.fillcolor('white')
.begin_fill()
t.circle(15)
t.end_fill()
t.penup()
t.goto(-30, 190)
t.pendown()
t.fillcolor('black')
t.begin_fill()
t.circle(5)
t.end_fill()
t.penup()
t.goto(30, 180)
t.pendown()
t.fillcolor('white')
t.begin_fill()
t.circle(15)
t.end_fill()
t.penup()
t.goto(30, 190)
t.pendown()
t.fillcolor('black')
t.begin_fill()
t.circle(5)
t.end_fill()
# 画嘴巴
t.penup()
t.goto(-50, 130)
t.pendown()
t.right(45)
t.circle(70, 90)
# 画鼻子
t.penup()
t.goto(0, 160)
t.pendown()
t.fillcolor('#FFA07A')
t.begin_fill()
t.circle(10)
t.end_fill()
# 画耳朵
t.penup()
t.goto(-80, 200)
t.pendown()
t.fillcolor('#F5DEB3')
t.begin_fill()
t.right(45)
t.circle(30, 90)
t.left(45)
t.circle(30, 90)
t.end_fill()
t.penup()
t.goto(80, 200)
t.pendown()
t.fillcolor('#F5DEB3')
t.begin_fill()
t.right(45)
t.circle(-30, 90)
t.left(45)
t.circle(-30, 90)
t.end_fill()
# 完成
turtle.done()
```
python卡通人物代码
以下是一个简单的Python代码,可以绘制一个卡通人物的螺旋线。
```python
import turtle
import time
# 创建一个海龟对象
t = turtle.Pen()
# 绘制螺旋线形状
for i in range(360):
t.forward(i)
t.left(59)
# 暂停程序一段时间
time.sleep(30)
```
这段代码使用了Python标准库中的turtle模块,通过控制海龟对象的移动和旋转来绘制螺旋线的形状。在循环中,每次向前移动的步长会逐渐增加,同时每次旋转的角度保持不变,从而形成了螺旋线的效果。最后,通过调用`time.sleep(30)`函数来暂停程序执行30秒。
阅读全文
相关推荐
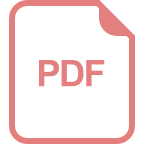
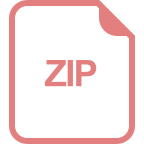
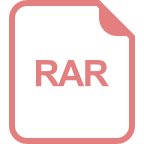


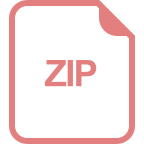
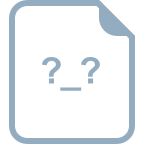
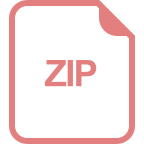
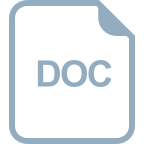
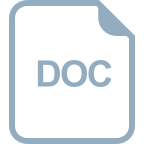
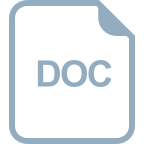




