C++耗时休眠时间函数
时间: 2024-10-22 20:01:22 浏览: 15
在C++中,可以使用`std::this_thread::sleep_for()`函数来使当前线程暂停一段时间。该函数允许指定时间段以指定的单位进行计算。具体地,你可以使用毫秒(ms)、秒(s)、分钟(m)、小时(h)等单位来指定休眠时间。
下面是一个使用`std::this_thread::sleep_for()`函数的示例代码:
```cpp
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "开始休眠...\n";
std::this_thread::sleep_for(std::chrono::seconds(5)); // 休眠5秒
std::cout << "休眠结束\n";
return 0;
}
```
在上面的示例中,`std::this_thread::sleep_for()`函数用于使当前线程休眠5秒钟。你可以根据需要将时间参数替换为不同的时间单位。注意,使用`std::this_thread::sleep_for()`函数可能会导致线程暂停一段时间,这可能会影响程序的性能和响应速度。在使用时应谨慎考虑。
相关问题
c++ future
C++11 引入了 std::future 用于表示异步操作的结果。std::future 对象可以用来获取异步操作的结果或者等待异步操作的完成。
当我们需要执行一个耗时的操作时,如果在主线程中执行,会阻塞主线程导致用户无法进行其他操作,为了避免这种情况,我们可以使用 std::async 来创建一个异步任务,std::async 函数会立即返回一个 std::future 对象,可以通过该对象来获取异步任务的执行结果或者等待异步任务的完成。
例如,下面的代码展示了如何使用 std::async 创建一个异步任务并获取结果:
```cpp
#include <iostream>
#include <future>
int main()
{
std::future<int> result = std::async([](){
// 模拟耗时操作
std::this_thread::sleep_for(std::chrono::seconds(2));
return 42;
});
// 获取异步任务的结果
int res = result.get();
std::cout << "The result is " << res << std::endl;
return 0;
}
```
上述代码中,通过 std::async 创建一个 lambda 表达式作为异步任务,该异步任务会休眠2秒钟后返回值 42。通过调用 std::future 的 get() 函数可以获取异步任务的结果。
c++ 异步线程示例
以下是一个C++的异步线程示例,使用了<future>库函数:
```c++
#include <iostream>
#include <future>
#include <chrono>
int main() {
// 异步执行一个函数
std::future<int> result = std::async([](){
std::this_thread::sleep_for(std::chrono::seconds(2)); // 模拟耗时操作
return 42;
});
// 主线程继续执行其他操作
std::cout << "Doing something else..." << std::endl;
// 等待异步操作完成并获取结果
int res = result.get();
// 输出结果
std::cout << "Result: " << res << std::endl;
return 0;
}
```
该示例中,我们使用了std::async函数异步执行了一个函数,该函数会休眠2秒钟,然后返回整数42。在主线程中,我们可以继续执行其他操作,而不必等待异步操作完成。最后,我们使用result.get()函数等待异步操作完成并获取结果,输出结果为“Result: 42”。
阅读全文
相关推荐
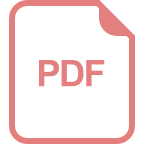
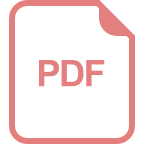
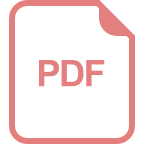
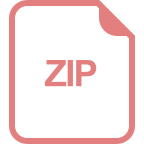
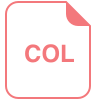
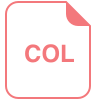
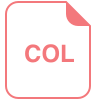
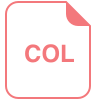
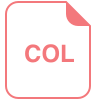
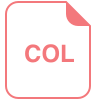
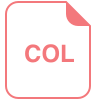
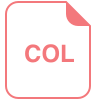
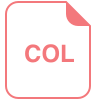
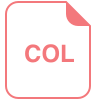
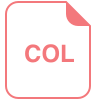

