python扫地机器人开发
时间: 2023-11-15 08:59:51 浏览: 167
Python扫地机器人开发是指使用Python语言编写程序,控制机器人进行扫地清洁的过程。在开发过程中,需要考虑机器人的移动、清洁范围、清洁时间等因素,以实现高效的清洁效果。通常,开发者需要使用传感器等硬件设备来获取机器人的位置信息,并根据算法控制机器人的移动和清洁。Python作为一种高级编程语言,具有易学易用、代码简洁等特点,因此在扫地机器人开发中得到了广泛应用。
相关问题
ros扫地机器人仿真
### 关于ROS扫地机器人仿真实现
#### 构建仿真环境
为了实现扫地机器人的仿真,可以采用ROS-Gazebo作为主要平台。该组合提供了丰富的工具集来模拟物理世界中的行为以及传感器数据[^1]。
#### 安装依赖包
确保安装了必要的软件包,比如`irobotcreate2ros`项目包含了针对iRobot Create 2型号的接口节点,虽然缺少某些特定类型的感应器支持如悬崖检测(cliff)和碰撞(bumper),但对于基本运动控制已经足够[^2]。
```bash
git clone https://github.com/CentroEPiaggio/irobotcreate2ros.git ~/catkin_ws/src/
cd ~/catkin_ws && catkin_make
source devel/setup.bash
```
#### 创建自定义ROS Node
按照教学材料指示创建一个新的ROS包用于开发漫游者(wanderer)逻辑——即让机器人持续前进直到遇到障碍物再调整方向继续前行的行为模式[^3]:
```xml
<launch>
<!-- 启动Gazebo并加载模型 -->
<include file="$(find gazebo_ros)/launch/empty_world.launch"/>
<!-- 加载机器人描述参数 -->
<param name="robot_description" command="$(find xacro)/xacro '$(find irobotcreate2ros)/urdf/create_2.urdf.xacro'" />
<!-- 插入机器人到场景中 -->
<node pkg="gazebo_ros" type="spawn_model" args="-param robot_description -urdf -model create2" />
</launch>
```
#### 实施清扫路径规划算法
除了简单的避障行走外,还可以加入更复杂的覆盖式清洁路线设计。这通常涉及到全局地图构建与维护、目标点选取及局部路径优化等方面的工作。
```python
import rospy
from nav_msgs.msg import Odometry
from geometry_msgs.msg import Twist
class CoveragePlanner(object):
def __init__(self):
self._cmd_pub = rospy.Publisher('/cmd_vel', Twist, queue_size=10)
def execute_plan(self):
rate = rospy.Rate(10)
while not rospy.is_shutdown():
twist_msg = Twist()
# 这里放置具体的移动指令计算
self._cmd_pub.publish(twist_msg)
rate.sleep()
if __name__ == '__main__':
try:
rospy.init_node('coverage_planner')
planner = CoveragePlanner()
planner.execute_plan()
except rospy.ROSInterruptException as e:
pass
```
通过上述步骤,在ROS框架下完成了一个基础版本的扫地机器人仿真应用。当然实际产品还需要考虑更多细节和技术挑战,但这提供了一个良好的起点[^4]。
扫地机器人路径规划算法ros
### ROS 中扫地机器人的路径规划算法
#### 路径覆盖策略的重要性
路径覆盖算法作为扫地机器人技术的关键组成部分,确保了设备能够在给定区域内高效移动并完成清扫工作。在ROS环境下开发的项目展示了如何利用节点实现虚拟清洁任务中的路径覆盖方案[^1]。
#### 随机碰撞寻路方法及其局限性
尽管许多商用产品依赖于简单的随机碰撞机制来进行导航,但由于缺乏优化后的路径规划能力,这些产品的实际表现往往不尽如人意。不同品牌间存在的性能差距主要源于各自所使用的软件算法质量不一[^2]。
#### 基于ROS的经典案例分析
为了帮助理解和实践这一领域内的概念和技术细节,有一个开源项目提供了完整的源代码以及详细的文档说明。该项目不仅包含了用于展示路径覆盖过程的小乌龟仿真模型(turtlesim),还深入探讨了差速驱动控制原理的应用场景。对于希望深入了解ROS平台下自动清扫解决方案的人来说,这无疑是一个宝贵的学习资源[^3]。
```python
import rospy
from geometry_msgs.msg import Twist, PoseStamped
from nav_msgs.msg import Odometry
from tf.transformations import euler_from_quaternion
import math
class PathPlannerNode(object):
def __init__(self):
self.publisher = rospy.Publisher('/cmd_vel', Twist, queue_size=10)
self.pose_subscriber = rospy.Subscriber("/odom", Odometry, self.update_pose)
self.rate = rospy.Rate(10) # 10hz
self.goal_position_x = None
self.goal_position_y = None
def update_pose(self,msg):
orientation_q=msg.pose.pose.orientation
_,_,yaw=euler_from_quaternion([orientation_q.x,
orientation_q.y,
orientation_q.z,
orientation_q.w])
position=msg.pose.pose.position
self.current_position=(position.x,position.y,yaw)
def move_to_goal(self,x,y):
goal_reached=False
while not goal_reached and not rospy.is_shutdown():
twist_msg=Twist()
if abs(x-self.current_position[0])<0.1 \
and abs(y-self.current_position[1])<0.1:
twist_msg.linear.x=0.0
twist_msg.angular.z=0.0
self.publisher.publish(twist_msg)
break
angle_to_target=math.atan2((y-self.current_position[1]),
(x-self.current_position[0]))
angular_error=angle_to_target-self.current_position[2]
linear_speed=min(math.sqrt(pow((x-self.current_position[0]),2)+
pow((y-self.current_position[1]),2)),0.5)*0.8
twist_msg.linear.x=linear_speed
twist_msg.angular.z=max(min(angular_error*4,-0.5),0.5)
self.publisher.publish(twist_msg)
self.rate.sleep()
if __name__=="__main__":
try:
rospy.init_node('path_planning_example')
planner=PathPlannerNode()
planner.move_to_goal(-2.0,-2.0)# Example target coordinates.
except rospy.ROSInterruptException as e:
pass
```
阅读全文
相关推荐










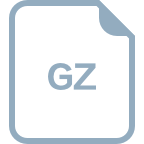

