c++将二进制字符串转换成十进制数
时间: 2024-10-27 10:07:29 浏览: 96
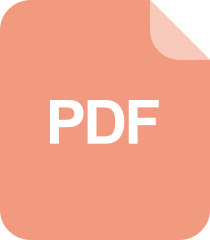
C++实现十六进制字符串转换为十进制整数的方法
在C++中,将二进制字符串转换成十进制数可以使用`std::stoi`函数,但是这个函数默认只能处理基于字符集的数字,对于纯二进制字符串(如"1010"),需要先手动将其转换为字符串形式。下面是一个示例:
```cpp
#include <string>
#include <sstream>
std::string binary_str = "1010";
int decimal_num;
// 将二进制字符串转换为10进制字符串
std::stringstream ss(binary_str);
ss >> std::hex; // 需要使用十六进制转义,因为二进制无法直接解析
decimal_num = std::stoi(ss.str(), nullptr, 16);
std::cout << "Decimal number from binary string: " << decimal_num << std::endl;
```
这里首先使用`std::stringstream`将二进制字符串转换到`std::hex`格式,然后通过`std::stoi`转换为十进制数。注意`nullptr`作为第二个参数,表明我们不关心错误提示的位置,`16`作为第三个参数指定基数为16(十六进制)。
阅读全文
相关推荐
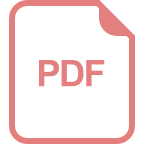


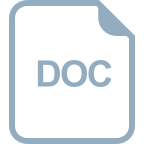







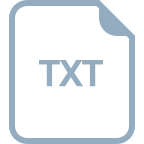




