使用tensorflow,灯笼检测并完成代码编写,性能评价
时间: 2024-10-10 08:09:22 浏览: 32
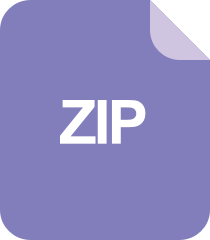
漂亮的动态飘花灯笼特效代码
在TensorFlow中,使用深度学习进行物体检测,如灯笼检测,通常会采用基于卷积神经网络(CNN)的对象检测模型,比如YOLO(You Only Look Once)或Faster R-CNN等。这里我会简述一个基本步骤:
首先,你需要安装TensorFlow库以及相关的深度学习框架,例如TensorFlow Object Detection API(基于SSD或Faster R-CNN)。
```python
pip install tensorflow tensorflow-object-detection-api
```
然后,下载预训练的模型,并选择一个适合灯笼检测的数据集,如PASCAL VOC或Custom Dataset,进行数据准备和标注。
接下来,使用`model_main.py`文件中的示例脚本创建配置文件,设置模型参数:
```python
config_path = 'path_to_your_config_file.config'
checkpoint_dir = 'path_to_pretrained_checkpoint/model.ckpt'
```
编写代码进行模型加载和预测:
```python
import cv2
from object_detection.utils import label_map_util
from object_detection.utils import visualization_utils as viz_utils
def detect_lanterns(image_path):
# 加载标签映射和类别索引
label_map = label_map_util.load_labelmap(config_path)
categories = label_map_util.convert_label_map_to_categories(label_map, max_num_classes=90, use_display_name=True)
category_index = label_map_util.create_category_index(categories)
# 加载模型并指定路径
detection_model = detection_model_lib.load_model(config_path, checkpoint_dir)
# 读取图像并进行检测
image = cv2.imread(image_path)
image_np = np.expand_dims(np.array(image), axis=0)
output_dict = detection_model.predict(image_np)
# 可视化结果
image_with_detections = viz_utils.visualize_boxes_and_labels_on_image_array(
image,
output_dict['detection_boxes'][0].numpy(),
(output_dict['detection_classes'][0].numpy() + label_map_util.start_id).astype(int),
output_dict['detection_scores'][0].numpy(),
category_index,
use_normalized_coordinates=True,
max_boxes_to_draw=20,
min_score_thresh=.5
)
plt.figure(figsize=(12, 8))
plt.imshow(cv2.cvtColor(image_with_detections, cv2.COLOR_BGR2RGB))
detect_lanterns('path_to_your_input_image.jpg')
```
性能评价通常是通过指标如平均精度(mAP)来进行的,它结合了召回率和精确度。TensorFlow Object Detection API通常会在训练过程中自动计算这些指标。如果你需要在测试阶段评估,可以使用`metrics_impl.compute_map()`函数。
阅读全文
相关推荐
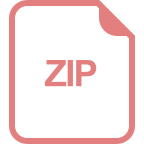

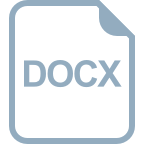
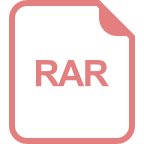
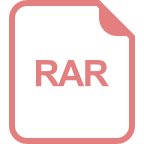
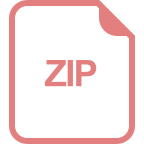
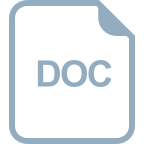
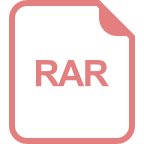
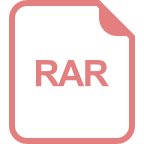
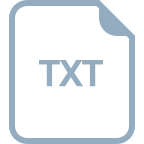