5.使用IDE创建一个Python工程,导入(import)Tensorflow/PyTorch、NumPy和MatPlotlib库,编写并运行一个Python程序,使用CPU进行大量线性运算,分析运行速度。
时间: 2024-09-23 20:16:39 浏览: 154
创建一个Python工程通常通过集成开发环境(IDE),例如PyCharm、VS Code或Jupyter Notebook。以下是使用PyCharm创建一个简单的Python项目,并导入TensorFlow、PyTorch、NumPy和Matplotlib库的步骤:
1. **安装必要的库**:
- 打开PyCharm,点击菜单栏的 "File" -> "New" -> "Project",选择 "Python"。
- 安装所需的库:在设置窗口中,点击 "Project Interpreter",然后点击 "+" 添加库。搜索并安装`tensorflow`, `torch`, `numpy` 和 `matplotlib`。
2. **新建文件并导入库**:
- 创建一个新的`.py`文件,如`linear_operation.py`。
```
import tensorflow as tf
import torch
import numpy as np
import matplotlib.pyplot as plt
```
3. **编写线性运算代码**:
使用TensorFlow和PyTorch做大量线性运算示例:
```python
# TensorFlow 示例
a = tf.random.uniform((10000, 10000))
b = tf.random.uniform((10000, 10000))
start_time = time.time()
c = tf.matmul(a, b)
tf_time = time.time() - start_time
# PyTorch 示例
torch_a = torch.randn(10000, 10000).cuda()
torch_b = torch.randn(10000, 10000).cuda()
start_time = time.time()
torch_c = torch.matmul(torch_a, torch_b)
torch_time = time.time() - start_time
# NumPy 示例
np_a = np.random.rand(10000, 10000)
np_b = np.random.rand(10000, 10000)
start_time = time.time()
np_c = np.dot(np_a, np_b)
np_time = time.time() - start_time
# 分析运行速度
print(f"TensorFlow CPU time: {tf_time} seconds")
print(f"PyTorch GPU time (CPU fallback): {torch_time} seconds")
print(f"NumPy CPU time: {np_time} seconds")
```
注意这里对于GPU加速,我们假设了有CUDA可用,如果在CPU上运行,`torch.matmul`会自动切换到CPU。
4. **运行程序**:
点击工具栏的 "Run" 或者按 F5 键来执行这个脚本,它会打印出每个库进行线性运算的时间。
阅读全文
相关推荐









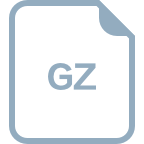








