c++调用qml显示图片
时间: 2023-10-20 13:03:00 浏览: 224
在C++代码中调用QML显示图片是可以通过以下步骤实现的:
1. 首先,在C++代码中引入QtQuick模块,包括QGuiApplication、QQmlApplicationEngine和QObject等必要的头文件。
2. 创建一个QGuiApplication对象,并执行应用程序。
```cpp
int main(int argc, char *argv[])
{
QCoreApplication::setAttribute(Qt::AA_EnableHighDpiScaling);
QGuiApplication app(argc, argv);
// ...
return app.exec();
}
```
3. 创建一个QQmlApplicationEngine对象,并加载QML文件。
```cpp
QQmlApplicationEngine engine;
engine.load(QUrl(QStringLiteral("qrc:/main.qml")));
```
4. 在QML文件中,使用Image组件来显示图片。为了让C++代码能够访问该Image组件的属性,需要使用QObject和QQmlContext。
```qml
import QtQuick 2.15
import QtQuick.Controls 2.15
ApplicationWindow {
visible: true
width: 640
height: 480
Image {
id: image
source: "path/to/image.png"
// ...
}
// Signal and slot to update image source
signal updateImageSource(string source)
Connections {
target: cppObject
onUpdateImageSource: {
image.source = source
}
}
}
```
5. 在C++代码中,通过QObject和QQmlContext获取Image对象,然后更新其source属性来改变显示的图片。
```cpp
QObject *rootObject = engine.rootObjects().first();
QObject *imageObject = rootObject->findChild<QObject*>("image");
QQmlContext *context = engine.rootContext();
context->setContextProperty("cppObject", this);
QString imagePath = "new/path/to/image.png";
QMetaObject::invokeMethod(imageObject, "updateImageSource",
Q_ARG(QVariant, imagePath));
```
通过以上步骤,即可在C++代码中成功调用QML显示图片。
阅读全文
相关推荐



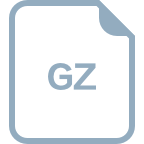













