C语言求模解年份为闰年,简单化
时间: 2024-11-05 14:08:54 浏览: 2
在C语言中,判断一个年份是否为闰年的简单算法通常基于以下规则:普通年能被4整除的是闰年,但是世纪年(即百年整数的年份)需满足能被400整除才是闰年。这个过程可以用取模运算符`%`来简化。以下是判断函数的一个基本示例:
```c
#include <stdio.h>
int is_leap_year(int year) {
// 如果年份能被4整除但不是世纪年,或者能被400整除,则是闰年
if ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0))
return 1; // 1表示闰年
else
return 0; // 0表示平年
}
int main() {
int year;
printf("请输入一个年份:");
scanf("%d", &year);
if(is_leap_year(year))
printf("%d年是闰年。\n", year);
else
printf("%d年不是闰年。\n", year);
return 0;
}
```
相关问题
C语言求模解年份为闰年,简单化,不用函数调用
在C语言中,判断一个年份是否为闰年的简单算法不需要专门定义函数,可以嵌套条件判断直接完成。根据公历规定,能被4整除的年份一般是闰年,但是世纪年份(即百位数不为0的年份)需要满足以下两个条件之一才是闰年:
1. 能被400整除;
2. 或者不能被100整除。
下面是简单的代码实现:
```c
#include <stdio.h>
int is_leap_year(int year) {
if (year % 4 == 0) {
if (year % 100 == 0) {
return (year % 400 == 0); // 如果是世纪年,检查能否被400整除
} else {
return 1; // 年份能被4整除但不是世纪年,则是闰年
}
} else {
return 0; // 否则不是闰年
}
}
int main() {
int year;
printf("请输入一个年份:");
scanf("%d", &year);
if (is_leap_year(year)) {
printf("%d年是闰年。\n", year);
} else {
printf("%d年不是闰年。\n", year);
}
return 0;
}
```
c语言求指定年份的第n天日期
### 回答1:
下面是一个简单的 C 语言程序,用于计算给定年份的第 n 天所对应的日期。程序假定每个月有 30 天。你可以根据需要进行修改以适应实际情况。
```c
#include <stdio.h>
int main()
{
int year, day, month, days_in_month, day_in_year;
printf("Enter year: ");
scanf("%d", &year);
printf("Enter day of the year: ");
scanf("%d", &day);
month = 1;
days_in_month = 30;
day_in_year = 0;
while (day > days_in_month && month <= 12) {
day -= days_in_month;
month++;
days_in_month = 30;
}
day_in_year += day;
printf("Date: %d-%d-%d\n", year, month, day_in_year);
return 0;
}
```
在这个程序中,我们首先输入年份和第 n 天。然后,我们初始化月份为 1,每个月的天数为 30,一年中的天数为 0。
接下来,我们使用一个循环来递增月份和减少天数,直到我们找到了正确的月份。我们假定每个月都有 30 天,但在实际情况下,每个月的天数是有所不同的。因此,你需要根据实际情况进行修改。
当我们找到了正确的月份时,我们计算出在该月中的天数(即 day_in_year),然后输出日期。
### 回答2:
要求使用C语言编写一个程序来找到指定年份的第n天对应的日期。我们可以使用C语言中的结构体来表示日期。首先,我们需要定义一个包含年、月、日的结构体,如下所示:
```
struct Date {
int year;
int month;
int day;
};
```
接下来,我们可以编写一个函数来计算指定年份的第n天对应的日期。函数的输入参数包括年份和天数,返回值为一个日期结构体。
```c
#include <stdio.h>
#include <stdbool.h>
bool isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return true;
} else {
return false;
}
}
struct Date getNthDate(int year, int n) {
struct Date date;
int daysOfMonth[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (isLeapYear(year)) {
daysOfMonth[1] = 29;
}
date.year = year;
date.month = 1;
date.day = 0;
while (n > 0) {
int remainingDays = daysOfMonth[date.month-1] - date.day;
if (n > remainingDays) {
n -= remainingDays;
date.month++;
date.day = 0;
} else {
date.day += n;
break;
}
}
return date;
}
int main() {
int year, n;
printf("请输入年份和天数:");
scanf("%d %d", &year, &n);
struct Date result = getNthDate(year, n);
printf("第%d天对应的日期为:%d年%d月%d日", n, result.year, result.month, result.day);
return 0;
}
```
以上就是使用C语言编写一个求指定年份的第n天日期的程序。程序首先判断给定的年份是否为闰年,然后根据不同月份的天数计算并更新日期。最后,程序输出计算得到的日期。
### 回答3:
要编写一个C语言程序来求指定年份的第n天的日期,首先需要了解一些基本的知识。
1. 闰年判断:
- 如果年份能被4整除,但不能被100整除,则为闰年。
- 如果年份能被400整除,则也是闰年。
2. 月份天数:
- 1、3、5、7、8、10和12月有31天。
- 4、6、9和11月有30天。
- 2月平年有28天,闰年有29天。
3. 算法思路:
- 首先,判断给定的年份是否为闰年。如果是,则将2月的天数设为29,否则为28。
- 然后,根据给定的天数n,逐个减去每个月的天数,直到n不够减时,即找到了对应的月份。
- 最后,根据剩下的天数n以及当前的月份,求得日期。
下面是一个简单的C语言代码示例:
```c
#include <stdio.h>
int isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return 1;
} else {
return 0;
}
}
void findDate(int year, int n) {
// 判断是否为闰年
int isLeap = isLeapYear(year);
int daysInMonth[13] = {0, 31, 28 + isLeap, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
// 逐个减去月份的天数
int month = 1;
while (n > daysInMonth[month]) {
n -= daysInMonth[month];
month++;
}
// 输出日期
printf("%d年第%d天是%d月%d日\n", year, n, month, n);
}
int main() {
int year, n;
printf("请输入年份和天数:");
scanf("%d %d", &year, &n);
findDate(year, n);
return 0;
}
```
以上是一个简单的C语言代码示例,用于求指定年份的第n天的日期。用户需要输入年份和天数,程序将计算出对应的日期并输出。
阅读全文
相关推荐
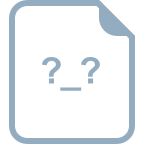
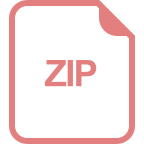
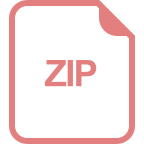
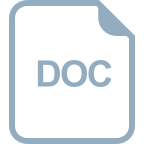
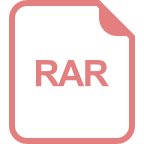
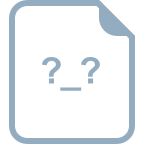
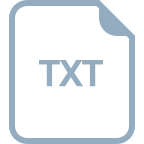
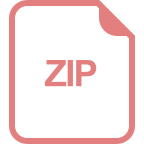
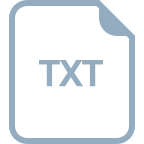
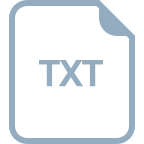
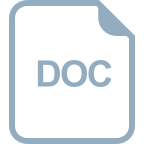
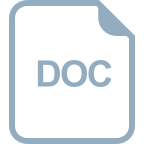
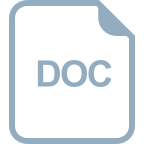
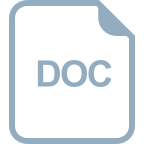
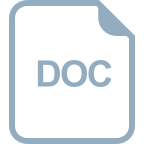
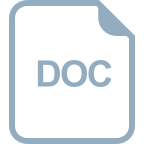
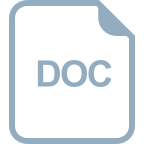